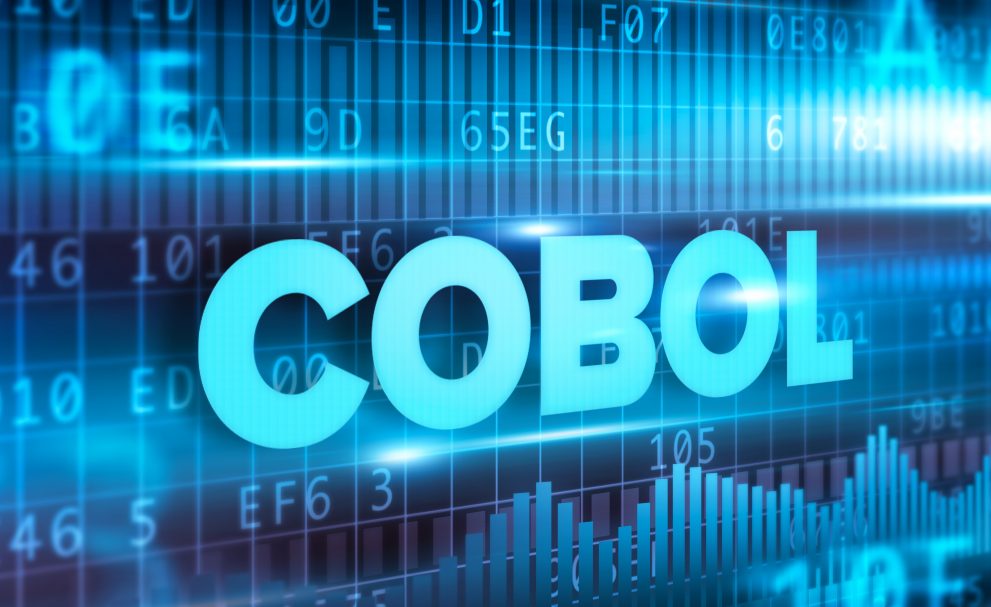
Any I-O operations on a file, there are chances that it may fail. We need to have COBOL FIle Error Handling. COBOL itself does not automatically take corrective action, we have to choose whether our program should continue running after an error or not. The severity of the error has to be defined in the program design stage. Let’s assume that we don’t have any file error handling. In such case, if READ operation is done and end of file is reached, the program will be terminated immediately with the error “End of file” Some techniques to handle certain I-O errors are:-
COBOL File Error Handling
- There are certain error handling clauses are provided by COBOL which can be used to handle file errors.
- AT END is used to handle error when read reaches to the end of file
- INVALID KEY is used to handle error when record is not found for specified key and thus cause execution of imperative statement.
FILE STATUS clause and File-Status Key
- In this technique, we can assign file-status to the file in the SELECT clause and verify the file status after every I-O and ensure it has permitted values. If not, then abnormally end the program with some display statement to make debugging easier.
COBOL File Error Handling – FILE STATUS CODES
The optional FILE STATUS clause specifies a data item that contains a file status code after any I-O statement (except RETURN) is applied to the file. Your program can contain USE procedures that examine the values of such data items and perform accordingly.
- FILE STATUS codes are checked in program to ensure last I-O is successful.
- It is two-byte in length and can be capture using working storage item defined as PIC X(2).
- The first byte indicates the general category whereas second byte indicates the specific type of error message under that category.
The first digit of a file status code indicates one of the following:
- The I-O operation was successful.
- An AT END condition occurred.
- An INVALID KEY condition occurred.
- A permanent error occurred.
- A logical error occurred.
- An implementation-defined condition occurred.
More than one file status code can apply to a situation (for example, a program can try to REWRITE a record that is too large and also fail to have a READ before the REWRITE). In this case, the file status code reflects the first error detected. The reason is that when the program detects one error, it does not continue to check for additional errors.
Term | Definition |
EOF | End of file. The program attempted to read a record following the last record in the file. |
AT END | An AT END condition caused a sequential READ statement to fail. |
INVALID KEY | One of the categories of file status codes. A code in this category means that an I-O operation failed for one of these reasons: 1. A duplicate key existed. 2. A boundary violation occurred. 3. The record sought was not found. 4. A sequence error occurred. This applies to indexed files only. |
Permanent Error | A category of file status code that indicates a problem accessing a permanent file. For example, an I-O statement failed due to an error that precluded further processing of the file. |
Logic Error | A category of file status code that indicates a problem with program logic. A code in this category means that an I-O statement failed for one of the following reasons: 1. An improper sequence of I-O statements was performed on the file. 2. A user-defined limit was violated. The record size is an example. |
COBOL File Error Handling
File Status Code | First Byte | Second byte | Description |
Successful | 0 | Successful OPEN/READ/WRITE operation | |
0 | Successful Completion | ||
2 | A duplicate Key condition exists, this is alright since this condition exists only when DUPLICATES has been specified for an AIX Possible causes: For a READ statement, the key value for the current key is equal to the value of that same key in the next record in the current key of reference. For a WRITE or REWRITE statement, the record just written created a duplicate key value for at least one alternate record key for which duplicates are allowed. | ||
4 | Invalid fixed length record – The length of the record being processed does not conform to the fixed file attributes for that file. | ||
5 | The referenced optional file is not present at the time the OPEN statement is executed. | ||
6 | Attempted to write to a file that has been opened for input. | ||
7 | Sequential files only. For an OPEN or CLOSE statement with the REEL/UNIT phrase the referenced file is a non-reel/unit medium. | ||
8 | Attempted to read from a file opened for output. | ||
9 | No room in directory or directory does not exist. | ||
AT END | 1 | When AT END condition encounters | |
0 | End of File encountered – No next logical record exists. You have reached the end of the file. | ||
2 | Attempted to open a file that is already open. | ||
3 | File not found. | ||
INVALID KEY | 2 | When INDEX key related error encounters | |
0 | Invalid Key for KSDS or RRDS | ||
1 | Out of sequence condition during sequential insertion – Sequentially accessed files only. Indicates a sequence error. The ascending key requirements of successive record key values has been violated, or, the prime record key value has been changed by a COBOL program between successful execution of a READ statement and execution of the next REWRITE statement for that file. | ||
2 | A duplicate key condition exists, no duplicates are allowed – Indexed and relative files only. Indicates a duplicate key condition. Attempt has been made to store a record that would create a duplicate key in the indexed or relative file OR a duplicate alternate record key that does not allow duplicates. | ||
3 | No record found – Indicates no record found. An attempt has been made to access a record, identified by a key, and that record does not exist in the file. Alternatively a START or READ operation has been tried on an optional input file that is not present. | ||
4 | KSDS/RRDS boundary violation, SHR (4 4) – Relative and indexed files only. Indicates a boundary violation. Possible causes: Attempting to write beyond the externally defined boundaries of a file Attempting a sequential WRITE operation has been tried on a relative file, but the number of significant digits in the relative record number is larger than the size of the relative key data item described for the file. | ||
Permanent Error | 3 | Permanent Open Error | |
0 | Input/Output physical error – The I/O statement was unsuccessfully executed as the result of a boundary violation for a sequential file or as the result of an I/O error, such as a data check parity error, or a transmission error. | ||
4 | ESDS boundary violation, SHR (4 4) – The I/O statement failed because of a boundary violation. This condition indicates that an attempt has been made to write beyond the externally defined boundaries of a sequential file. | ||
5 | While performing OPEN File and file is not present – An OPEN operation with the I-O, INPUT, or EXTEND phrases has been tried on a non-OPTIONAL file that is not present. | ||
7 | OPEN file with wrong mode – An OPEN operation has been tried on a file which does not support the open mode specified in the OPEN statement. | ||
8 | Tried to OPEN a Locked file – An OPEN operation has been tried on a file previously closed with a lock. | ||
9 | OPEN failed because of conflicting file attributes – A conflict has been detected between the fixed file attributes and the attributes specified for the file in the program. This is usually caused by a conflict with record-length, key-length, key-position or file organization. Other possible causes are: Alternate indexes are incorrectly defined. The Recording Mode is Variable or Fixed or not defined the same as when the file was created.. | ||
Logic Error | 4 | Logic error in OPEN/CLOSE/REWRITE | |
1 | Tried to OPEN a file that is already open. | ||
2 | Tried to CLOSE a file that is not OPEN. | ||
3 | Tried to REWRITE without READing a record first – Files in sequential access mode. The last I/O statement executed for the file, before the execution of a DELETE or REWRITE statement, was not a READ statement. | ||
4 | Tried to REWRITE a record of a different length – A boundary violation exists. Possible causes: Attempting to WRITE or REWRITE a record that is larger than the largest, or smaller than the smallest record allowed by the RECORD IS VARYING clause of the associated file Attempting to REWRITE a record to a file and the record is not the same size as the record being replaced. | ||
6 | Tried to READ beyond End-of-file – A sequential READ operation has been tried on a file open in the INPUT or I-O mode but no valid next record has been established. | ||
7 | A READ or START operation has been tried on a file not opened INPUT or I-O. | ||
8 | A WRITE operation has been tried on a file not opened in the OUTPUT, I-O, or EXTEND mode, or on a file open I-O in the sequential access mode | ||
9 | Tried to DELETE or REWRITE to a file that was not opened I-O | ||
Implementation – Defined | 9 | Implementation defined | |
0 | Error condition, catch all if error does not fit category above | ||
1 | Password or authorization failed – For VSAM only. | ||
2 | Logical error, usually out of sequence commands, sometimes invalid operation for file type, trying to read from an output file, etc. – For VSAM only. | ||
3 | Resource not available – For VSAM only. | ||
4 | For VSAM with CMPR2 compiler-option only: No file position indicator for sequential request. | ||
5 | For VSAM only. Invalid or incomplete file information. | ||
6 | No file identification – For VSAM under MVS: No DD statement specified for this file. FOR VSAM and SAM under VSE: No DLBL statement specified for this file. | ||
7 | OPEN successful and file integrity verified – For VSAM only. | ||
8 | File is Locked – OPEN failed | ||
9 | Record Locked – Record access failed |