Optimize Outer Join queries is a powerful tool for retrieving data from multiple tables. However, if not used judiciously, they can lead to performance issues. This blog post will explore tips and tricks to optimize outer join queries for better performance. Let’s dive into practical examples and explanations.
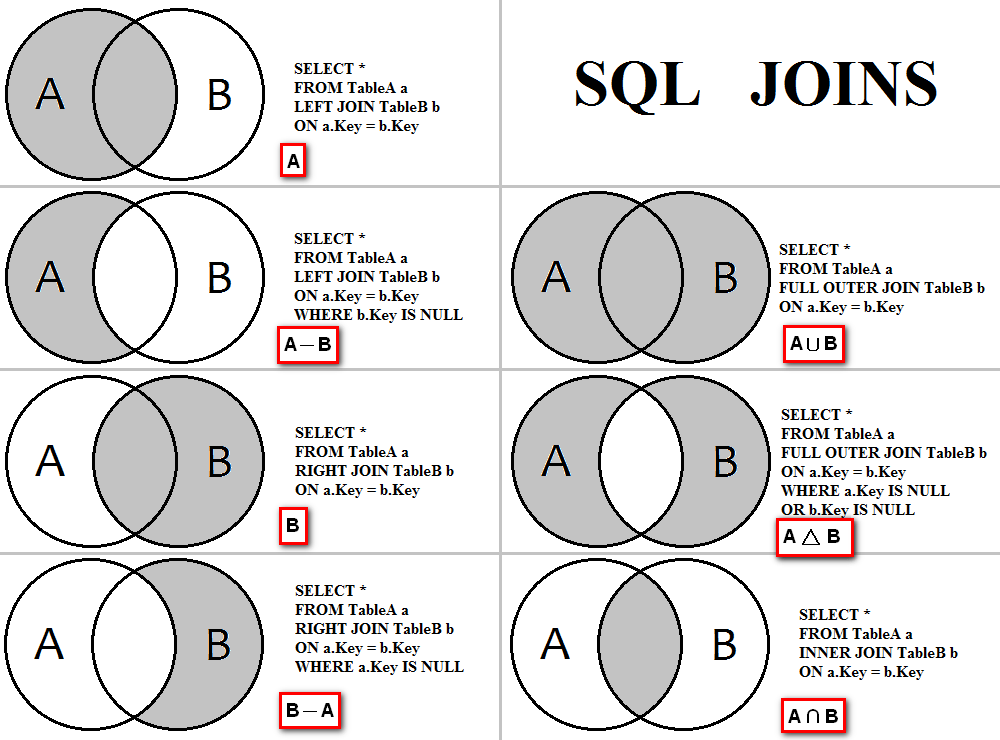
Choose the Right Join Type
Full outer joins, while inclusive, can be resource-intensive. If you only need data from one table with potential matches in another, opt for left or right outer joins to reduce processing overhead. Understand your data and choose between LEFT and RIGHT JOIN based on the table with more essential information. This can sometimes improve query performance.
Example: Efficient Left Outer Join SELECT c.customer_id, c.name, o.order_date FROM customers c LEFT OUTER JOIN orders o ON c.customer_id = o.customer_id /* Indexed column */ WHERE o.order_date IS NULL /* Filter after the join */
Use INNER JOINs Where Appropriate
Before opting for an outer join, evaluate whether an INNER JOIN can serve your purpose. INNER JOINs generally perform better than OUTER JOINs because they return only the matching rows.
Example: Using INNER JOIN instead of LEFT JOIN SELECT * FROM table1 t1 INNER JOIN table2 t2 ON t1.id = t2.id;
Filter Rows Early with WHERE Clause
Apply filtering conditions as early as possible using the WHERE clause. This reduces the number of rows involved in the join, improving performance.
Example: Applying WHERE condition before the OUTER JOIN SELECT * FROM table1 t1 LEFT JOIN table2 t2 ON t1.id = t2.id WHERE t2.status = ‘Active';
Be Cautious with DISTINCT
Avoid using DISTINCT unnecessarily. It can lead to performance degradation, especially in combination with OUTER JOINs. Ensure you genuinely need distinct values before using it.
Example: Minimize the use of DISTINCT SELECT DISTINCT t1.id, t1.name FROM table1 t1 LEFT JOIN table2 t2 ON t1.id = t2.id;
Limit Result Set with ROW_NUMBER()
When dealing with large result sets, consider using ROW_NUMBER() to limit the number of rows returned.
Example: Using ROW_NUMBER() to limit result set SELECT * FROM ( SELECT t1.*, ROW_NUMBER() OVER (ORDER BY t1.id) AS row_num FROM table1 t1 LEFT JOIN table2 t2 ON t1.id = t2.id ) AS numbered WHERE row_num <= 100; SELECT c.customer_id, c.name, o.order_date FROM customers c LEFT OUTER JOIN ( SELECT * FROM orders o WHERE order_date > '2023-01-01' /* Filter within subquery */ ) ON c.customer_id = o.customer_id
Minimize Unnecessary Data
- Select only the columns you truly need via the SELECT clause to reduce data processing and transfer.
- Consider using summary tables or views to pre-aggregate data before joining, especially for large datasets.
Optimize Outer Join Conditions
- Craft efficient join conditions using indexed columns for quick matching.
- Avoid using functions or expressions within join conditions, as they can hinder performance.
- Use explicit join syntax (JOIN…ON) for clarity and potential performance gains.
Consider Materialized Views
For frequently used complex queries involving outer joins, consider using materialized views. These precomputed views can significantly boost performance.
Example: Creating a materialized view CREATE MATERIALIZED VIEW mv_example AS SELECT * FROM table1 t1 LEFT JOIN table2 t2 ON t1.id = t2.id;
Index Properly
Ensure that columns used in join conditions are indexed. Indexing accelerates the search for matching rows, enhancing query speed.
- Ensure indexes are present on columns involved in join conditions for faster matching.
- Rebuild or reorganize indexes periodically to maintain their efficiency.
Utilize Database-Specific Optimization Features
Take advantage of DB2’s query optimization tools, such as the Visual Explain utility, to analyze query plans and identify potential bottlenecks.
Remember:
- Thoroughly test your queries to gauge performance gains and identify any unintended side effects.
- Monitor query execution plans to pinpoint potential bottlenecks and adjust your optimization strategies accordingly.
Conclusion
Optimizing outer join queries involves a combination of thoughtful design, proper indexing, and efficient use of SQL clauses. By applying these tips and tricks, you can enhance the performance of your queries and create a more responsive database system. Always remember to analyze and test the impact of optimizations on your specific database and data characteristics.