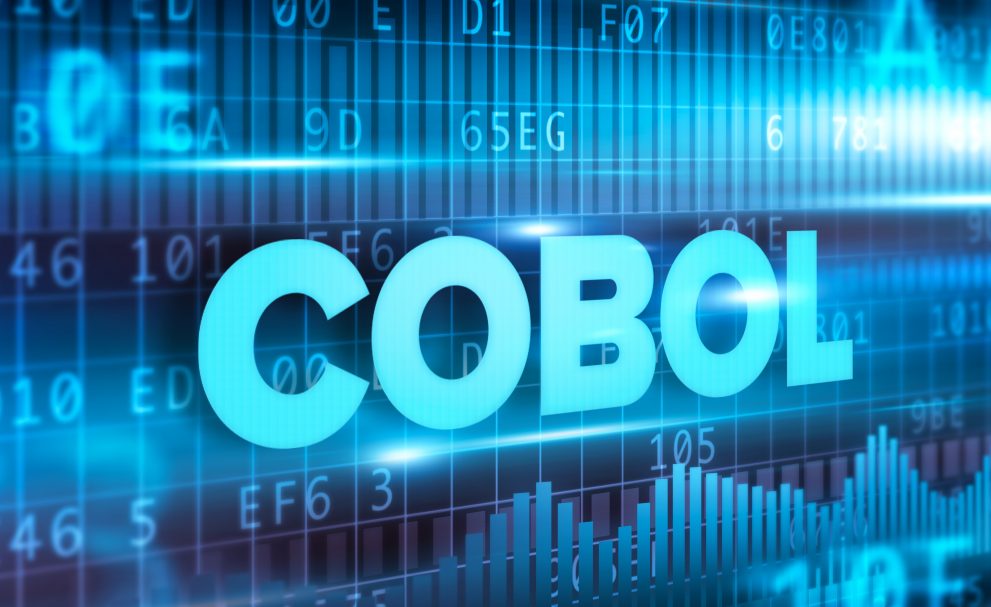
Conditional expressions identify conditions that are tested to enable the object program to select between alternate paths of control depending upon the truth value of the condition. Conditional expressions may be used in the EVALUATE, IF, PERFORM, and SEARCH statements. Conditional expressions have a value of “TRUE” or “FALSE”. Conditional expressions can either be simple or complex. There are various types of Conditional statements in Cobol :
- Relational condition.
- Sign condition.
- Class condition.
- Condition-name condition.
- Negated condition.
- Compound condition.
- If condition.
- If-else condition.
- Nested If condition.
Conditional Expressions : Relational Conditions
A relation condition specifies a comparison of two operands, each of which may be a data item or a literal. Comparison between operand/s or literal/s can be done using relational operators i.e. < , > , < = , >= , ==. We can either use the particular relational symbol or we can spell it out in words.Â
VALUE-1 IS [NOT] { GREATER THAN } VALUE-2 { > } { LESS THAN } { < } { EQUAL TO } { = } { <> } { GREATER THAN OR EQUAL TO} { >= } { LESS THAN OR EQUAL TO } { <= }
Meaning | Operators |
Greater than | IS GREATER THAN IS > IS NOT <= IS NOT LESS THAN OR EQUAL TO |
Equal to | IS EQUAL TO IS = |
Less than | IS LESS THAN IS < IS NOT >= IS NOT GREATER THAN OR EQUAL TO |
Greater than or equal | IS NOT LESS THAN IS NOT < IS >= IS GREATER THAN OR EQUAL TO |
Not equal to | IS NOT EQUAL TO IS NOT = IS <> |
Less than or equal | IS NOT GREATER THAN IS NOT > IS <= IS LESS THAN OR EQUAL TO |
IS not like | IS NOT LIKE |
Comparison of numeric operands
- For operands whose class is numeric, a comparison is made with respect to the algebraic value of the operands. The size of the operands (in terms of the number of digits) is not significant.Â
- Zero is a unique value regardless of sign. Comparison is allowed regardless of the USAGE of the operands.Â
- Unsigned operands are considered positive or zero. Index data items are compared as if they were numeric operands.
Comparison of nonnumeric operands
- For non-numeric operands, or one numeric and one non-numeric operand, a comparison is made with respect to the program’s collating sequence of characters.
- If one of the operands is numeric, it must be an integer data item or an integer literal. The numeric operand is treated as though it were moved to an alphanumeric data item of the same size as the numeric data item. This alphanumeric item is then used in the comparison. A non-integer numeric operand may not be compared to a non-numeric operand.
- When the comparison is performed, the shorter operand (if any) is treated as though it were extended on the right with spaces to make the operands of equal size.Â
- Characters are then compared in corresponding positions starting from the left end and continuing until either a pair of unequal characters is encountered or the right end of the operand is reached. If all the characters are the same, then the operands are considered equal. Otherwise, the operand with the smaller character in the first unequal pair is considered less than the other operand.
Conditional Expressions : Class Condition
The class condition tests whether or not an operand contains a particular type of data. The format of a class condition is:
Arithmetic-expression IS [NOT] {POSITIVE} {NEGATIVE} {ZERO }
An arithmetic expression is POSITIVE if it is greater than zero, NEGATIVE if it is less than zero. If the NOT option is specified, the truth value of the test is reversed.
Conditional Expressions : Complex Conditions
A complex condition is formed by combining conditions (either simple or complex) with logical connectors (AND and OR) or by negating these conditions with logical negation (NOT). The truth value of a complex condition depends on the interaction of the logical operators and their component conditions.
The logical operators and their meanings are:
Operator | Meaning |
AND | true when both components true |
OR | true when either component true |
NOT | true when condition false |
A condition is negated by the logical operator NOT, which reverses the truth value of the condition to which it is applied. In other words, a negated condition is true when its component condition is false, and is false when its component condition is true. The format of a negated condition is:
NOT condition
Conditional Expressions : Combined conditions
A combined condition results from connecting conditions with one of the logical operators AND or OR. The general format is:
condition { {AND} condition } ... {OR }
In the general format, the condition may be any of the following:
- a simple condition
- a negated simple condition
- a combined condition
- a negated combined condition; that is, a “NOT” followed by a combined condition enclosed in parentheses
Parentheses may be used in complex conditions to alter the rules of evaluation. Parentheses must be made in matched pairs and must be placed in such a way that the enclosed symbols constitute a well-defined condition.
The truth value of a combined condition using AND is true only when both component conditions are true. The truth value of a combined condition using OR is true when either or both of the component conditions are true.
Abbreviated Combined Relation Conditions
When simple or negated simple conditions are combined in a consecutive sequence, the relation conditions may be abbreviated. You can do this by either:
- omitting the subject of the relation condition (the left-hand component condition).
- omitting both the subject and the relational operator.
The format for an abbreviated combined relation condition is:
condition { {AND} [NOT] [relation] object } ... {OR }
Within a sequence of relation conditions, both of the above forms of abbreviation may be used. When you use such abbreviations, it is as if the last preceding stated subject was inserted in the place of the omitted subject, and the last stated relational operator was inserted in the place of the omitted relational operator. The insertion of an omitted subject or relational operator terminates once a complete simple condition is encountered within a complex condition. Except for the source of the initial relation condition, no parentheses may appear in the sequence of abbreviated conditions.
If the word NOT is used in an abbreviated combined relation condition, it has the following meaning:
- If the word immediately following NOT is GREATER, “>”, LESS, “<“, EQUAL, “<=”, “>=”, or “=”, then the NOT participates as part of the relational operator.
- Otherwise, the NOT is interpreted as a logical operator and the implied insertion of the subject or relational operator results in a negated relation condition.
The following are examples of abbreviated combined relation conditions and their expanded equivalents:
A > B AND NOT < C (A > B) AND (A NOT < C) A NOT = B OR C (A NOT = B) OR (A NOT = C) NOT A = B OR C (NOT (A = B)) OR (A = C) NOT (A > B OR < C) NOT ((A > B) OR (A < C))
Order of Evaluation
A condition is evaluated according to the following hierarchy:
- Components contained in parentheses are evaluated first. Evaluation always progresses from the most to the least deeply nested components.
- NOT conditions are evaluated next. Thus NOT A OR B is equivalent to (NOT A) OR B.
- AND conditions are evaluated next.
- OR conditions are evaluated next.
- Conditions are evaluated from left to right.
Here are some examples of equivalent conditions:
NOT A AND B OR C AND D ((NOT A) AND B) OR (C AND D) NOT A AND B AND C ((NOT A) AND B) AND C A AND B OR NOT (C OR D) (A AND B) OR (NOT (C OR D)) NOT ( NOT A OR B OR C ) NOT ( ((NOT A) OR B) OR C )
Evaluation of a condition halts as soon as its truth value is determined. For example, in conditions A AND B, condition `B’ would not be evaluated if `A’ were false.