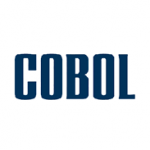
Using indexes to address a table is more efficient than using subscripts since the index already contains the displacement from the start of the table and does not have to be calculated at run time.
Subscripts, on the other hand, contain an occurrence number that must be converted to a displacement value at run time before it can be used.
When using subscripts to address a table, use a binary signed data item with eight or fewer digits (for example, using PICTURE S9(8) COMP) for the data item). This will allow full word arithmetic to be used during the calculations. Additionally in some cases, using four or fewer digits for the data item may also offer some added reduction in CPU time since half word arithmetic can be used.
Indexing
You create an index by using the INDEXED BY phrase of the OCCURS clause to identify an index-name.
For example, INX-A in the following code is an index-name:
05 TABLE-ITEM PIC X(8) OCCURS 10 INDEXED BY INX-A.
The compiler calculates the value contained in the index as the occurrence number (subscript) minus 1, multiplied by the length of the table element. Therefore, for the fifth occurrence of TABLE-ITEM, the binary value contained in INX-A is (5 – 1) * 8, or 32.
You can use the USAGE IS INDEX clause to create an index data item, and can use an index data item with any table. For example, INX-B in the following code is an index data item:
77 INX-B USAGE IS INDEX.. . . SET INX-A TO 10 SET INX-B TO INX-A. PERFORM VARYING INX-A FROM 1 BY 1 UNTIL INX-A > INX-B DISPLAY TABLE-ITEM (INX-A) . . . END-PERFORM.
The index-name INX-A is used to traverse table TABLE-ITEM above. The index data item INX-B is used to hold the index of the last element of the table. The advantage of this type of coding is that calculation of offsets of table elements is minimized, and no conversion is necessary for the UNTIL condition.
You can use the SET statement to assign to an index data item the value that you stored in an index-name, as in the statement SET INX-B TO INX-A above. For example, when you load records into a variable-length table, you can store the index value of the last record into a data item defined as USAGE IS INDEX. Then you can test for the end of the table by comparing the current index value with the index value of the last record. This technique is useful when you look through or process a table.
You can increment or decrement an index-name by an elementary integer data item or a nonzero integer literal, for example:
SET INX-A DOWN BY 3
The integer represents a number of occurrences. It is converted to an index value before being added to or subtracted from the index.
Subscripting
Initialize the index-name by using a SET, PERFORM VARYING, or SEARCH ALL statement. You can then use the index-name in SEARCH or relational condition statements. To change the value, use a PERFORM, SEARCH, or SET statement.
Because you are comparing a physical displacement, you can directly use index data items only in SEARCH and SET statements or in comparisons with indexes or other index data items. You cannot use index data items as subscripts or indexes.
The lowest possible subscript value is 1, which references the first occurrence of a table element. In a one-dimensional table, the subscript corresponds to the row number.
You can use a literal or a data-name as a subscript. If a data item that has a literal subscript is of fixed length, the compiler resolves the location of the data item.
When you use a data-name as a variable subscript, you must describe the data-name as an elementary numeric integer. The most efficient format is COMPUTATIONAL (COMP) with a PICTURE size that is smaller than five digits. You cannot use a subscript with a data-name that is used as a subscript. The code generated for the application resolves the location of a variable subscript at run time.
You can increment or decrement a literal or variable subscript by a specified integer amount. For example:
TABLE-COLUMN (SUB1 – 1, SUB2 + 3)
You can change part of a table element rather than the whole element. To do so, refer to the character position and length of the substring to be changed. For example:
01 ANY-TABLE. 05 TABLE-ELEMENT PIC X(10) OCCURS 3 TIMES VALUE "ABCDEFGHIJ" MOVE "??" TO TABLE-ELEMENT (1) (3 : 2).
The MOVE statement in the example above moves the string ‘??’ into table element number 1, beginning at character position 3, for a length of 2 characters.
Performance Considerations for Indexes Vs Subscripts
- Using binary data items (COMP) to address a table is 56% slower than using indexes
- Using decimal data items (COMP-3) to address a table is 426% slower than using indexes
- Using DISPLAY data items to address a table is 680% slower than using indexes
COBOL Blog: Click Here IBM Reference:Click Here