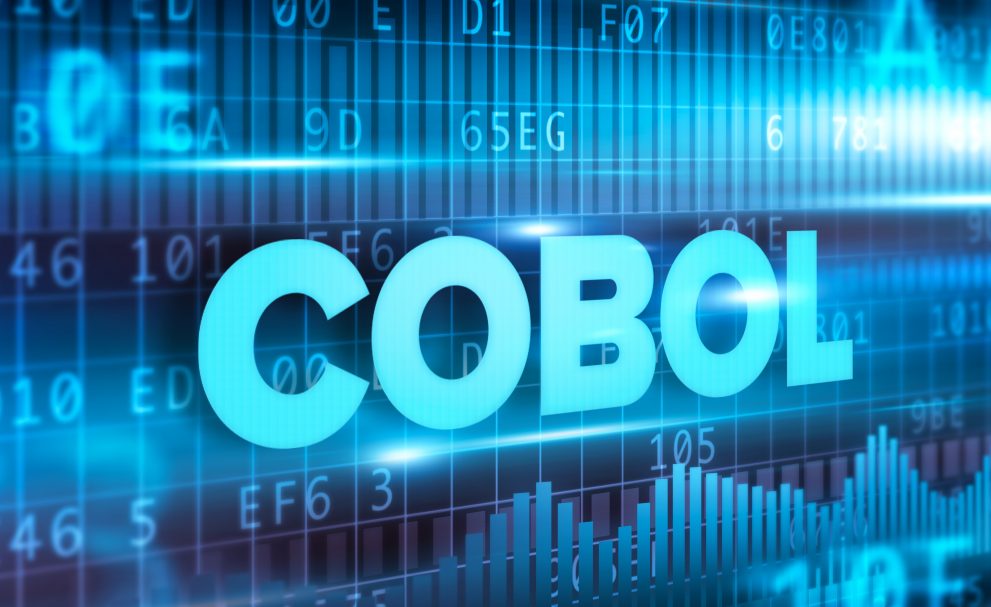
COBOL Intrinsic Functions provides many ways to manipulate data. These are a set of functions that return values from a specific algorithm on input arguments. Intrinsic Functions are available for mathematics, character manipulation, date/time and statistics, and financial work. These functions are elementary data items that will return a numeric (whole or decimal) or an alphanumeric. The structure of the call is keyword FUNCTION followed by the specific function followed by any required or optional arguments.
Intrinsic Functions: UPPER-CASE, LOWER-CASE
The intrinsic functions UPPER-CASE returns a character string that is the same length as the argument specified, with each lowercase letter replaced by the corresponding uppercase letter. The argument must be alphabetic or alphanumeric and must be at least one character in length.
MOVE FUNCTION UPPER-CASE(WS-STATE-NAME) TO STATE-NAME.
The LOWER-CASE function returns a character string that is the same length as the argument specified with each uppercase letter replaced by the corresponding lowercase letter.
The argument must be alphabetic or alphanumeric and must be at least one character in length.
MOVE FUNCTION LOWER-CASE(WS-STATE-NAME) TO STATE-NAME.
Intrinsic Functions: LENGTH, TRIM, REVERSE
The length function gets the length of non-numeric fields so that you can find out the length of the field.
COMPUTE LEN-FLD = FUNCTION LENGTH(FLD-IN)
The TRIM function returns the given string with any leading and trailing blanks removed, or the given string with any leading and trailing specified characters removed.
MOVE FUNCTION TRIM(FLD-A) TO FLD-B.
The TRIML function returns the given string with any leading blanks removed or the given string with any leading specified characters removed.
MOVE FUNCTION TRIML(FLD-A) TO FLD-B.
The TRIMR function returns the given string with any trailing blanks removed, or the given string with any trailing specified characters removed.
MOVE FUNCTION TRIMR(FLD-A) TO FLD-B.
The REVERSE function returns a character string that is exactly the same length as the argument, whose characters are exactly the same as those specified in the argument, except that they are in reverse order.
The argument must be alphabetic or alphanumeric and must be at least one character in length.
MOVE FUNCTION REVERSE(FLD-A) TO FLD-B.
Arithmetic Functions:
- The MAX function returns the content of the argument that contains the maximum value.
- The MIN function returns the content of the argument that contains the minimum value.
- The SUM function returns a value that is the sum of the arguments.
- The MOD function result is an integer with as many digits as the shorter of argument-1 and argument-2.
- The REM function returns a numeric value that is the remainder of argument-1 divided by argument-2.
- The SQRT function returns a numeric value that approximates the square root of the argument specified.
- The FACTORIAL function returns an integer that is the factorial of the argument specified.
Example:
COMPUTE WS-MAX = FUNCTION MAX(FLD-A FLD-B FLD-C FLD-D). COMPUTE WS-MIN = FUNCTION MIN(FLD-A FLD-B FLD-C FLD-D). COMPUTE WS-TOTAL = FUNCTION SUM(FLD-A FLD-B FLD-C FLD-D). COMPUTE WS-MOD = FUNCTION MOD(FLD-A FLD-B). COMPUTE WS-REM = FUNCTION REM(FLD-A FLD-B). COMPUTE WS- SQRT = FUNCTION SQRT(FLD-A). COMPUTE WS- FACTORIAL = FUNCTION FACTORIAL(FLD-A).
Intrinsic Functions: NUMVAL, NUMVAL-C, INTEGER
The NUMVAL function returns the numeric value represented by the alphanumeric character string specified in an argument. The function strips away any leading or trailing blanks in the string, producing a numeric value that can be used in an arithmetic expression.
COMPUTE ANS-WS = FUNCTION NUMVAL(FLD-A).
The NUMVAL-C function returns the numeric value represented by the alphanumeric character string (numeric edited field) specified as the argument. Any optional currency sign specified or any optional commas preceding the decimal point are stripped away, producing a numeric value that can be used in an arithmetic expression. The NUMVAL-C function may not be specified under the following conditions:
- More than one CURRENCY SIGN clause is specified within the program
- The WITH PICTURE SYMBOL phrase is specified in a CURRENCY SIGN clause
- A lowercase letter is specified as the currency symbol
COMPUTE ANS-WS = FUNCTION NUMVAL-C("$23,678.86 CR").
The INTEGER function returns the greatest integer value that is less than or equal to the argument. If the decimal is greater than 5, it will round. If you want to truncate, use INTEGER-PART.
COMPUTE INT-ANS-WS = FUNCTION INTEGER(12.123). returns 12 COMPUTE WS-INT-VALUE = FUNCTION INTEGER(45.7). returns 46 COMPUTE WS-INT-VALUE = FUNCTION INTEGER-PART(123.456). returns 123 COMPUTE INT-ANS-WS = FUNCTION INTEGER-PART(13.98). returns 13
Intrinsic Functions: DATE
Julian date : This format of date is a combination of year plus a relative day number within the year, which is more correctly called an ordinal date. A typical example is 2013348 in the format YYYYDDD. This is equivalent to a calendar date of December 14th 2013.
Gregorian date : This format of date corresponds to any of the industry standard date formats supported by DB2. For example, the International Organization for Standardization (ISO) format is CCYY-MM-DD. 2013-12-14 is equivalent to the calendar date December 14th 2013.
INTEGER-OF-DATE
The INTEGER-OF-DATE function converts a date in the Gregorian calendar from standard date form (YYYYMMDD) to integer date form.
FUNCTION INTEGER-OF-DATE(WS-DATE-YYYYMMDD)
INTEGER-OF-DAY
The INTEGER-OF-DAY function converts a date in the Gregorian calendar from Julian date form (YYYYDDD) to integer date form.
FUNCTION INTEGER-OF-DAY(WS-DATE-YYYYDDD)
DATE-OF-INTEGER
The DATE-OF-INTEGER function converts a date in the Gregorian calendar from integer date form to standard date form (YYYYMMDD).
FUNCTION DATE-OF-INTEGER(WS-DATE-YYYYMMDD)
DAY-OF-INTEGER
The DAY-OF-INTEGER function converts a date in the Gregorian calendar from integer date form to Julian date form (YYYYDDD).
FUNCTION DAY-OF-INTEGER(WS-DATE-YYYYDDD)
EXTRACT-DATE-TIME
The EXTRACT-DATE-TIME function returns part of a date, time, or timestamp item.
COMPUTE WS-INTEGER-1 = FUNCTION EXTRACT-DATE-TIME(DATE-3 MONTHS). COMPUTE WS-INTEGER-1 = FUNCTION EXTRACT-DATE-TIME(DATE-3 '%m'). MOVE FUNCTION EXTRACT-DATE-TIME (DATE-2 '%m/%d') to ALPHANUM-1.
CURRENT-DATE
The CURRENT-DATE function returns a 21-character alphanumeric value that represents the calendar date, time of day, and time differential from Greenwich Mean Time provided by the system on which the function is evaluated. The format is yyyymmddhhmmsstt+hhmm.
WS-DATE = FUNCTION CURRENT-DATE
DATEVAL
DATEVAL intrinsic function can be used to convert a non-date into a date field. The first argument to the function is the non-date to be converted, and the second argument specifies the date format. The second argument is a literal string with a specification similar to that of the date pattern in the DATE FORMAT clause.
Syntax - Function DATEVAL(Date-Copied "YYXXXX")
DATEVAL intrinsic function can also be used in a comparison expression to convert a literal to a date field, and the output from the intrinsic function will then be treated as either a windowed or expanded date field to ensure a consistent comparison.
Date-Due = Function DATEVAL(051220 "YYXXXX") Date-Due = Function DATEVAL(20200101 "YYYYXXXX")
Examples of Intrinsic Functions
QUESTION: WHAT IS THE DIFFERENCE IN DAYS BETWEEN FEBRUARY 28, 2019 AND MARCH 2, 2019? COMPUTE DATE-DIFF = FUNCTION INTEGER-OF-DATE (20190302) -FUNCTION INTEGER-OF-DATE (20120228). Answer- 3
QUESTION: WHAT WILL BE THE DATE 365 DAYS IN THE PAST AND FUTURE FROM AUGUST 15,1999? COMPUTE FUTURE-DATE = FUNCTION DATE-OF-INTEGER (FUNCTION INTEGER-OF-DATE (19990815)- 365). COMPUTE FUTURE-DATE = FUNCTION DATE-OF-INTEGER (365 + FUNCTION INTEGER-OF-DATE (19990815)). Answer - AUGUST 14, 2000
QUESTION: CALCULATE THE DAY OF THE WEEK FOR A GIVEN DATE? 01 DATE-VAR PIC 9(04) VALUE ‘20200101’ COMPUTE INT-DATE = FUNCTION INTEGER-OF-DATE(DATE-VAR). COMPUTE DAY-NUM = FUNCTION MOD( INT-DATE 7 ). EVALUATE DAY-NUM WHEN 0 DISPLAY "SUN" WHEN 1 DISPLAY "MON" WHEN 2 DISPLAY "TUE" WHEN 3 DISPLAY "WED" WHEN 4 DISPLAY "THU" WHEN 5 DISPLAY "FRI" WHEN 6 DISPLAY "SAT" END-EVALUATE. Answer: WED
QUESTION: FIND IF THE YEAR IS A LEAP YEAR? 01 WS-YEAR PIC 9(04) VALUE ‘2020’ EVALUATE TRUE WHEN FUNCTION MOD (WS-YEAR 4) NOT ZERO WHEN FUNCTION MOD (WS-YEAR 100) ZERO AND FUNCTION MOD (WS-YEAR 400) NOT ZERO DISPLAY 'IT IS NOT A LEAP YEAR ' WS-YEAR WHEN OTHER DISPLAY 'IT IS A LEAP YEAR ' WS-YEAR END-EVALUATE Answer: IT IS A LEAP YEAR 2020
QUESTION: CONVERT GREGORIAN TO JULIAN DATE AND VICE VERSA 01 WS-GREGORIAN-DATE PIC 9(08) VALUE '20101202'. 01 WS-JULIAN-DATE PIC 9(07) VALUE '2010336'. COMPUTE WS-JULIAN-DATE = FUNCTION DAY-OF-INTEGER (FUNCTION INTEGER-OF-DATE (WS-GREGORIAN-DATE)) COMPUTE WS-GREGORIAN-DATE = FUNCTION DATE-OF-INTEGER (FUNCTION INTEGER-OF-DAY (WS-JULIAN-DATE)) Answer: 2010336 , 20101202