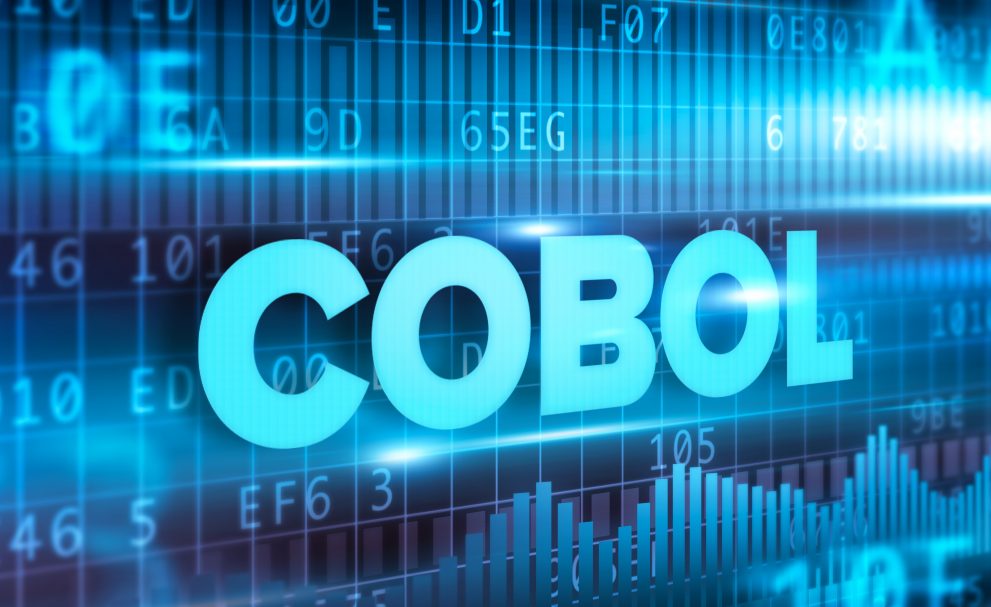
The COBOL MERGE statement combines two or more identically sequenced files on a set of specified keys. As part of the merge operation, it makes records available in their merged order to an output procedure or an output file. The records are made available following the actual merging of the files. The output procedure or the moving of records to an output file is considered part of the merge process. MERGE statements may appear anywhere within the PROCEDURE DIVISION except in the declarative portion or in an input or output procedure associated with a COBOL SORT or COBOL MERGE statement.
In the merge process, three kinds of files are used:-
- input-file: Two or more input files that are to be merged
- work-file: Temporary work file, which will be used during merge process The SD(sort description) entry for file should be defined in FILE SECTION
- output-file: File on which merged records are written
MERGE file-name-1 ON ASCENDING KEY data-name-1 [ON DESCENDING KEY data-name-1] [COLLATING SEQUENCE IS alphabet-name] USING file-name—2, file-name—3 …… {OUTPUT PROCEDURE IS procedure-name-1 thru procedure-name-2] GIVING file-name—4. SD work-file [RECORD CONTIANS integer CHARACTERS] [DATA RECORD IS file-rec-1]
COBOL MERGE Parameters
Parameter | Description |
file-name—1 | sort/merge file, and is described in a sort/merge file description (SD level) entry in the DATA DIVISION. |
data items described in records associated with file-name-1. Each may be qualified and may vary in length. None of these data names can be described by an entry either containing an OCCURS clause, or subordinate to an entry containing such a clause. If file-name-1 has more than one record description, then the data items represented by the data names need to be described in only one of the record descriptions. | |
alphabet-name | either EBCDIC, STANDARD-1, NATIVE, or an alphabet name as defined by you in the SPECIAL-NAMES paragraph of the ENVIRONMENT DIVISION. |
file-name-2 File-name-3 | files to be merged. These files must not be open at the time the MERGE statement is executed. Each must be a file described in an FD level file description in the DATA DIVISION. No more than one such file name can name a file from a multiple file reel. Any given file name can be used only once in any given MERGE statement The actual size of the logical record or records described for these files must be equal to the actual size of the logical record or records described for file-name-1. If the data descriptions of the elementary items that make up these records are not identical, it is your responsibility to describe the corresponding records in such a way as to cause an equal number of character positions to be allocated for the corresponding records. |
Procedure-name-1 | name of the first paragraph or section in an output procedure. |
Procedure-name-2 | name of the last paragraph or section in an output procedure. |
file-name-4 | names of output files. These files are subject to the same restrictions as file-name-2. |
The MERGE statement merges all records contained in the files named in the USING phrase. The files to be merged are automatically opened and closed by the merge operation with all implicit functions performed, such as the execution of any associated USE procedures. The files described by file-name-2 and file-name-3 must not be open when the MERGE verb is executed, and may not be opened or closed during an output procedure if one is specified.
Following the actual merging of the files, but before they have been closed, the merged records are released in the order in which they were merged. They are released to either the specified output procedure or the specified output file.
ASCENDING or DESCENDING Phrase
The results of a merge operation are predictable only when the records in the files to be merged are ordered as described in the ASCENDING or DESCENDING KEY phrase associated with the MERGE statement.
The data names following the word KEY are listed from left to right in order of decreasing significance. This decreasing significance is maintained from the KEY phrase to the KEY phrase. Thus, in the format shown, data-name-1 is the most significant, and each successive data name is the next most significant.
When the MERGE statement is executed, the records of file-name-2 and file-name-3 are merged in the specified order (ASCENDING or DESCENDING) using the most significant key data item. Within the records having the same value for the most significant key data item, the records are merged according to the next most significant key data item; this kind of merging continues until all key data items named in the MERGE statement have been used.
When the ASCENDING phrase is used, the merged records are in a sequence starting from the lowest value of the key data items and ending with the highest value.
When the DESCENDING phrase is used, the merged records are in a sequence from the highest value of the key data item to the lowest.
Merging takes place using the rules for the comparison of operands of a related condition. If all corresponding key data items of records to be merged are equal, the records are written to file-name-4, or returned to the output procedure, in the order that the input files are specified in the MERGE statement.
COLLATING SEQUENCE Phrase
The COLLATING SEQUENCE phrase allows you to specify what collating sequence to use in the merging operation. This phrase is optional. The program collating sequence is used if none is specified in the MERGE statement.
GIVING and OUTPUT PROCEDURE Phrases
- You must specify either the GIVING or OUTPUT PROCEDURE phrase in a MERGE statement.
- If you specify the GIVING phrase, all merged records are automatically written to one or more occurrences of file-name-4. Files named in the GIVING phrase can be sequential, relative, or indexed.
- If you use the OUTPUT PROCEDURE phrase, there are several rules you must follow in writing the procedure.
- The procedure must consist of one or more paragraphs or sections that appear contiguously in the source program, and that are not part of any other procedure.
Important consideration about MERGE
- All input files must be sequential files and must be sorted on merge keys.
- Each sd-rec-keys (shown in SORT syntax) identifies a field in the record of work-file, upon which the file will be sorted.
- When more than one sd-file-key is specified, the keys decrease in significance from left to right (leftmost key is most major key i.e. most significant and likewise rightmost key is least significant).
- It is possible to use both the words ASCENDING and DESCENDING in single SORT statement but for different keys. If all keys to be sorted in same order, then ASCENDING or DESCENDING needs to be mentioned only once before the most major key.
- The order of appearance of keys in SORT statement has no relevance in their position in the record.
- All kind of files must have same records size and have same position of the merge keys.
COBOL Merge performs the following operations
- Opens the work-file in I-O mode, input-files in the INPUT mode and output-file in the OUTPUT mode.
- Transfers the records present in the input-files to the work-file.
- Sorts the SORT-FILE in ascending/descending sequence by rec-key.
- Transfers the sorted records from the work-file to the output-file.
- Closes the input-file and the output-file and deletes the work-file.
Example: Let’s say there are two different classroom files containing student information and we want to create one merged file containing all students from both classes. This can be achieved using MERGE.
FILE-CONTROL. SELECT CLASSROOM-1 ASSIGN TO ROOM1. SELECT CLASSROOM-2 ASSIGN TO ROOM2. SELECT ALL-CLASSROOM ASSIGN TO ALLROOM. SELECT WORK-FILE ASSIGN TO WORKFL. FILE-SECTION. FD ROOM-1. 01 ROOM-1-REC PIC X(15). FD ROOM-2. 01 ROOM-2-REC PIC X(15). FD ALL-CLASSROOM. 01 ALL-CLASSROOM-REC PIC X(15). SD WORK-FILE. 01 WORK-REC. 05 STUDENT-ID-WRK PIC 9(05). 05 STUDENT-NAME-WRK PIC X(50). MERGE WORK-FILE ON ASCENDING KEY STUDENT-ID-WRK USING ROOM-1,ROOM-2 GIVINS ALL-CLASSROOM.
Sample of Merging with both ascending and descending with the procedure.
MERGE WORK-FILE ON ASCENDING KEY STUDENT-ID-WRK ON DESCENDING KEY STUDENT-NAME-WRK USING ROOM-1,ROOM-2 OUTPUT PROCEDURE IS PUT-RECORDS.