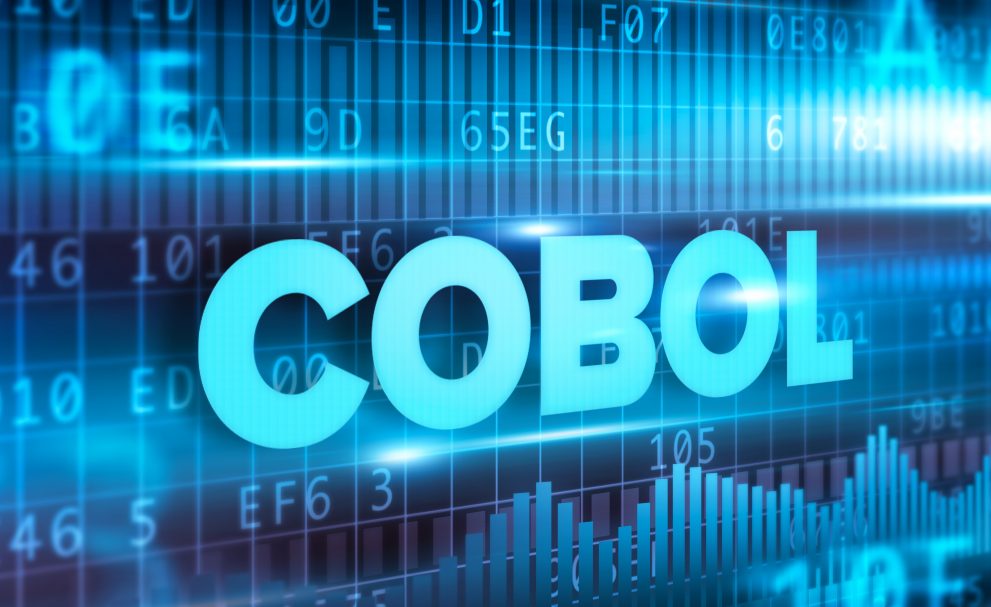
The collection of records belonging to the same entity is known as File. These records are stored permanently. File handling helps to organize these records in an ascending/descending order. It makes searching, accessing these records more easily and efficiently. The records are generally stored on a magnetic tape or a disk.
Advantages of File Handling
- It has unlimited storage and thus stores a large volume of data.
- It stores the data permanently on the device.
- It reduces the re-editing of data.
Disadvantages of File Handling
- It provides slow access.
- Cannot perform operations efficiently.
File handling verbs in COBOL helps to perform different desired operations on the files. These verbs are:
- OPEN
- CLOSE
- READ
- WRITE
- REWRITE
- START
- DELETE
File Operations Allowed based on Modes –
Permissible statements for sequential files
INPUT Mode | OUTPUT Mode | I-O Mode | EXTEND Mode | |
READ | X | X | ||
WRITE | X | X | ||
REWRITE | X |
Permissible statements for indexed and relative files
Sequential Access
Operation | INPUT Mode | OUTPUT Mode | I-O Mode | EXTEND Mode |
READ | X | X | ||
WRITE | X | X | ||
REWRITE | X | |||
START | X | X | ||
DELETE | X |
Random Access
Operation | INPUT Mode | OUTPUT Mode | I-O Mode | EXTEND Mode |
READ | X | X | ||
WRITE | X | X | ||
REWRITE | X | |||
START | ||||
DELETE | X |
Dynamic Access
Operation | INPUT Mode | OUTPUT Mode | I-O Mode | EXTEND Mode |
READ | X | X | ||
WRITE | X | X | ||
REWRITE | X | |||
START | X | X | ||
DELETE | X |
Below is a detailed description of these File Handling verbs.
OPEN: OPEN verb opens the file to perform further operations on it i.e. it makes the file available to perform any operation. You cannot perform any operation without opening the file, thus this must be the first operation that should be performed on a file. There are 4 modes in which a file can be opened:
- INPUT mode: It helps to read the records/data from a file.
- OUTPUT mode: It helps to write the records/data in a file.Files contain records: The file gets replaced by new data. File defined with a DD dummy card: Unpredictable results can occur.
- EXTEND mode: It helps to write the new record/data at the end of the file, i.e. it does not delete the previous records of the file, unlike the OUTPUT mode.
- I-O mode: It opens the file in INPUT as well as in OUTPUT mode.
Syntax:
OPEN {INPUT/OUTPUT/EXTEND/I-O} file_name_1[,file_name_2,...].
File Opening mode | File Status if Unsuccessful |
INPUT | Open is unsuccessful. (file status 35) |
INPUT (optional file) | Normal open; the first read causes the at end condition or the invalid key condition. (file status 05) |
I-O | Open is unsuccessful. (file status 35) |
I-O (optional file) | Open causes the file to be created. (file status 05) |
OUTPUT | Open causes the file to be created. |
EXTEND (optional file) | Open causes the file to be created. (file status 05) |
Example:
OPEN OUTPUT OP_FILE
CLOSE: The CLOSE statement terminates the processing of volumes and files.A CLOSE statement can be executed only for a file in open mode. A file should be closed after performing all the operations. CLOSE verb disables the link between the file and the program. After performing a close operation the file variables will no longer be available for performing the operation. If the file is in an open status and the execution of a CLOSE statement is unsuccessful, the EXCEPTION/ERROR procedure for the file is executed.
Syntax:
CLOSE file_name_1[,file_name_2,....].
Example:
CLOSE OP_FILE
READ: READ verb allows to read the records of a file. At once, only one record can be read into the file structure and after reading a record the file pointer is incremented by one. To read the records the file must be opened in either INPUT mode or in I-O mode. As soon as the file pointer reaches the end of the file the imperative statement written in the “AT END” clause is executed.
Syntax:
READ file-name [NEXT/PREVIOUS] RECORD [INTO identifier-1] AT END imperative statement NOT AT END imperative statement
END-READ.
Following is the syntax to write a record when the file access mode is random
READ file-name RECORD INTO ws-file-structure KEY IS rec-key INVALID KEY DISPLAY 'Invalid Key’ NOT INVALID KEY DISPLAY 'Record Details: ' ws-file-structure END-READ.
Example: READ INPT_FILE AT END MOVE ‘Y’ TO EOF.
KEY IS – It is used to access the records randomly by passing the key before READ. The KEY IS phrase can be specified only for indexed files. Data-name-1 must identify a record key associated with file-name-1. data-name-1 can be qualified however it can’t be subscripted.
NEXT RECORD – Reads the next record in the logical sequence of records. NEXT is optional when the access mode is sequential and has no effect on READ statement execution. Either the NEXT phrase or the PREVIOUS phrase must specify for files reading in dynamic access mode.
PREVIOUS RECORD – Reads the previous record in the logical sequence of records. PREVIOUS phrase applies to indexed and relative files with DYNAMIC access mode. Either the NEXT or PREVIOUS phrase must specify setting is used to determine which record to make available according to the following rules –
- If the file position indicator indicates that no valid previous record has been established, the READ is unsuccessful.
- If the file position indicator is positioned by the execution of an OPEN statement, the AT END condition occurs.
- If the file position indicator is established by a previous START statement, the first existing record in the file whose relative record number (if a relative file) or whose key value (if an indexed file) is less than or equal to the file position indicator is selected.
- If the file position indicator is established by a previous READ statement, the first existing record in the file whose relative record number (if a relative file) or whose key value (if an indexed file) is less than the file position indicator is selected.
Example: READ NEXT INPT_FILE AT END MOVE 'Y' TO WS-EOF-SW
OPEN INPUT INPT_FILE. PERFORM UNTIL WS-EOF='Y' READ INPT_FILE INTO WS-INPT-REC AT END MOVE 'Y' TO WS-EOF NOT AT END DISPLAY WS-INPT-REC END-READ END-PERFORM. CLOSE INPT_FILE.
The below example will read the record with Input Key = 5
MOVE 5 TO INPT-ID. READ INPT_FILE RECORD INTO WS-INPT-FILE KEY IS INPT-ID INVALID KEY DISPLAY 'Invalid Key' NOT INVALID KEY DISPLAY WS-INPT-FILE END-READ.
INVALID KEY phrase
Both the INVALID KEY phrase and an applicable EXCEPTION/ERROR procedure can be omitted. The invalid key condition can occur during the execution of a READ statement.
When an invalid key condition occurs, the READ is unsuccessful. If the INVALID KEY phrase is specified and condition occurred, control is transferred to the INVALID KEY imperative statement.
If the INVALID KEY phrase is not specified in the READ statement for a file and an applicable EXCEPTION/ERROR procedure exists, that procedure is executed. The NOT INVALID KEY phrase if specified is ignored.
Both the INVALID KEY phrase and the EXCEPTION/ERROR procedure can be omitted. If the invalid key condition does not exist, the INVALID KEY phrase is ignored if specified.
WRITE: WRITE verb allows writing the record into the file. At once only one record can be written into the file structure and after writing a record the file pointer is incremented by one, thus the records are written one after the other. To write the records into the file, the file must be opened into OUTPUT mode or I-O mode. If we want to write the values of identifier-1 into the file then the “FROM” clause is executed.
Syntax:
WRITE record-name [ FROM identifier-1].
Example:
WRITE OP_FILE
WRITE OP_FILE FROM WS-OUTPUT-REC
Controls positioning of the output record on the page.
Advancing Phrase: Control positioning of output records on the page.
Rules: –
- When the ADVANCING phrase is specified, the following rules apply –
- When BEFORE ADVANCING is specified, the line is printed before the page is advanced.
- When AFTER ADVANCING is specified, the page is advanced before the line is printed.
- When identifier-2 is specified, the page is advanced the number of lines equal to the identifier-2 value. identifier-2 must name an elementary integer data item. identifier-2 cannot name a date field.
- When integer is specified, the page is advanced the number of lines equal to the integer value.
- Integer or the value in identifier-2 can be zero.
- When PAGE is specified, the record is printed on the logical page BEFORE or AFTER the device is positioned to the next logical page. If PAGE has no meaning for the device used, then BEFORE or AFTER ADVANCING 1 LINE is provided.
- When the ADVANCING phrase is omitted, automatic line advancing is applicable as AFTER ADVANCING 1 LINE.
REWRITE: REWRITE verb helps to update an existing record, i.e. if the user wants to rewrite or make any changes to the existing record then use the REWRITE verb. To rewrite the record, the file must be opened in I-O mode. If the user wants to write the values of identifier-1 into the file then the “FROM” clause is executed.
Syntax:
REWRITE record-name [FROM identifier-1].
Example:
REWRITE OP_FILE
REWRITE OP_FILE FROM WS-OUTPUT-REC
START: If the user wants to start reading the record from a particular location then use the START verb. The file must be opened in I-O mode and the access mode of the file must be either SEQUENTIAL or DYNAMIC. If either the comparison is not satisfied by any key or the file is accessed from an undefined position then the “INVALID KEY” clause is executed.
KEY phrase
The KEY phrase is used to position the file position indicator at the record that satisfies the specified KEY value in the file. When the KEY phrase is not specified, KEY IS EQUAL is applied. If the KEY is specified with ‘less than’ or ‘less than or equal to’, the file position indicator is positioned to the last logical record that satisfies the comparison. For an indexed file, if the key has duplicate entries that satisfy the comparison, the file position indicator is positioned at the last record of the entries.
INVALID KEY phrase
If the comparison is not satisfied by any record in the file, an invalid key condition exists; the position of the file position indicator is undefined, and (if specified) the INVALID KEY imperative-statement is executed. Both the INVALID KEY phrase and an applicable EXCEPTION/ERROR procedure can be omitted.
Syntax:
START file-name [KEY IS {EQUAL TO/ = /GREATER THAN/ > /NOT LESS THAN/NOT < THAN} data-name] [INVALID KEY imperative statement].
Example:
START INPT_FILE KEY IS EQUAL STD-NO INVALID KEY DISPLAY "INVALID KEY". FILE-CONTROL. SELECT INPT_FILE ASSIGN TO DISK. ORGANIZATION IS INDEXED. ACCESS MODE IS DYNAMIC. RECORD KEY IS STD-NO. FILE STATUS IS WS-FS. FILE SECTION. FD INPT_FILE. 01 STD-REC. 02 STD-NO PIC 9(03). 02 STD-NAME PIC X(20). 02 STD-GENDER PIC X(07). 02 FILLER PIC X(50). PROCEDURE DIVISION. ……………… START INPT_FILE KEY EQUAL STD-NO. INVALID KEY DISPLAY 'NO STUDENT FOUND WITH GIVEN STD-NO' NOT INVALID KEY PERFORM READ-NEXT-RECS. ………..
DELETE: The DELETE statement removes a record from an indexed or relative file. DELETE is not applicable to sequential files as the record deletion is not possible. The file must be opened in I-O mode. If the access mode of the file is SEQUENTIAL then the INVALID KEY phrase should not be specified and the DELETE verb must be preceded with the READ statement on the file. If the user tries to delete the record which does not exist in the file then the “INVALID KEY” clause is executed. If the FILE STATUS clause is specified, the associated file status key is updated with the execution status of the DELETE statement. The file position indicator is not affected by the execution of the DELETE statement.
The records are deleted like below based on the access mode –
Sequential access mode – The previous READ statement must be successfully executed before deleting the statement. When the
Random or Dynamic access mode – Random or Dynamic access mode – When the DELETE statement is executed on indexed files, the system removes the record identified by the contents of the RECORD KEY data item. When the DELETE statement is executed on relative files, the system removes the record identified by the contents of the RELATIVE KEY data item for relative files. If the file does not contain a record trying to delete, an INVALID KEY condition exists. After the successful execution of DELETE statement and NOT INVALID KEY phrase specified, the imperative statements specified with NOT INVALID KEY are executed.
Once the record gets deleted, the below things happen –
- For indexed files, the space available for any new record and the key can be reused for record addition.
- For relative files, the space is available for a new record with the same RELATIVE KEY value.
Syntax:
DELETE file-name [ INVALID KEY imperative statement].
Example:
DELETE OP_FILE
DELETE OP_FILE INVALID KEY DISPLAY " WRONG KEY".
Conclusion
File handling in COBOL is a critical aspect of data processing applications. Understanding and effectively using file handling verbs empower COBOL programmers to interact with data stored in files seamlessly. Whether it’s reading records, updating information, or managing file connections, these verbs form the backbone of COBOL programs, contributing to the language’s enduring relevance in the business computing landscape.