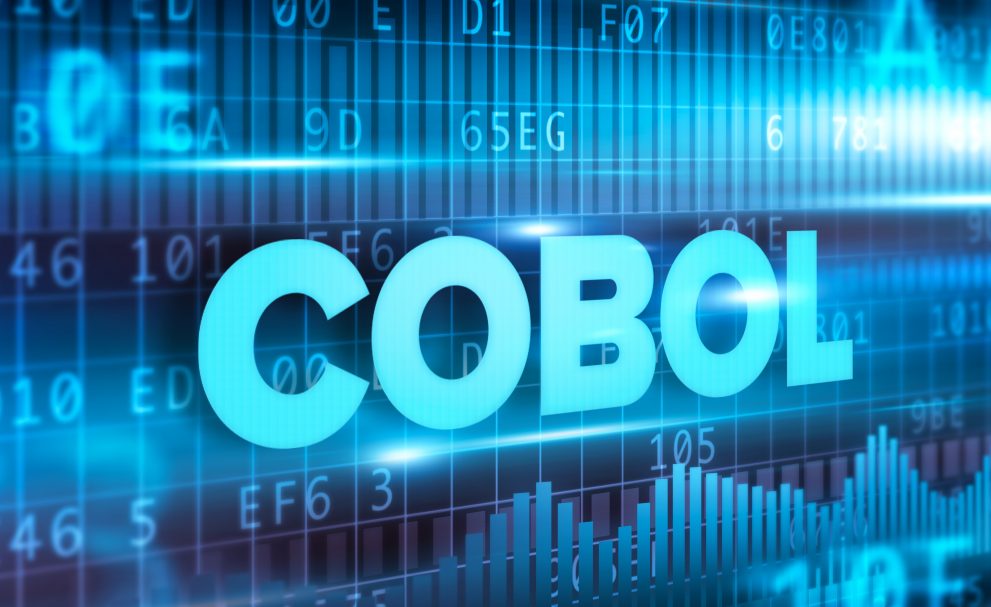
The FIND DURATION function is used to calculate duration between:
- Two dates
- A date and a timestamp
- Two times
- A time and a timestamp
- Two timestamps.
The FIND-DURATION function returns an integer in the form of complete units of the specified duration. Any rounding is done downwards. The calculation of durations includes microseconds.
The function type is integer.
The function result is a nine-digit integer. If the function result is larger than 9 digits (999,999,999), a machine check occurs.
FIND DURATION FORMAT
FUNCTION FIND-DURATION (argument-1 argument-2 argument-3 )
argument-1, argument-2
- Must be a date, time, or timestamp item.
- Argument-1 is subtracted from argument-2. The value returned is the number of complete units of argument-3. If argument-1 is later than argument-2, the result is negative. If argument-1 is earlier than argument-2, the result is positive.
argument-3
Is a keyword that represents a duration. The valid Find Duration keywords are:
- YEARS
- MONTHS
- DAYS
- HOURS
- MINUTES
- SECONDS
- MICROSECONDS
- PICOSECONDS
In order to determine the valid duration keywords, the following rules apply:
- If argument-1 or argument-2 is a date item, the duration specified must be consistent with a date.
- If argument-1 or argument-2 is a time item, the duration specified must be consistent with a time.
- If the returned value is not an integer, it is truncated. For example, the duration between March 17, 1997 and May 2, 1997 is 1.5 months. Since FIND-DURATION only returns an integer the .5 would be truncated, and the actual value returned would be 1.
- PICOSECONDS duration can only be requested when argument-1 and argument-2 are timestamp items.
Example:
COMPUTE integer-1 = FUNCTION FIND-DURATION (date-3 date-4 MONTHS). COMPUTE integer-1 = FUNCTION FIND-DURATION (timestamp-1 date-5 MONTHS).
ADD DURATION – COBOL Function
The ADD-DURATION function adds duration to a date, time, or timestamp item, and returns the modified item.
The function type is date-time.
The length of the returned value depends on the length of the date, time, or timestamp item specified in argument-1. The returned value will be truncated to the length of argument-1.
If a duration is added to a date item, the date returned must fall within a certain range:
- For 4-digit dates, the range must be 0001/01/01 through 9999/12/31
- For 2-digit dates, the range must be 0001/01/01 through 9999/12/31, but the year is truncated to 2 digits
- For a 3-digit year (a 1-digit century and a 2-digit year), the range must be 1900/01/01 through 2899/12/31 (the default). This range can be changed by specifying the DATTIM PROCESS statement option.
If a duration is added to a 2-digit date item, the range is the same as for a 4-digit year, but the year in the value returned is truncated to 2 digits.
ADD DURATION Format
FUNCTION ADD-DURATION (argument-1 argument-2 argument-3)
argument-1
- Must be date, time, or timestamp data item.
- Argument-1 is a data item containing a value to which a duration is added. The duration is specified in argument-2 and argument-3.
argument-2
Argument-2 is a keyword that represents a duration. The valid duration keywords are:
- YEARS
- MONTHS
- DAYS
- HOURS
- MINUTES
- SECONDS
- MICROSECONDS
- PICOSECONDS
The duration keyword must be consistent with argument-1. For example, the duration keywords most obey the following rules:
- YEARS, MONTHS, and DAYS can only be added to a date or timestamp item.
- HOURS, MINUTES, SECONDS, and MICROSECONDS can only be added to a time or timestamp item.
- PICOSECONDS can only be added to a timestamp item.
argument-3
- Must be an integer arithmetic expression. Argument-3 is the number of units of the duration, as specified in argument-2, that are to be added to argument-1.
- Argument-3 can be a negative integer, but the function only takes its absolute value. If argument-3 is longer than 9 digits, it is truncated.
- Argument-2 and argument-3 can be repeated. There should be no duplicate argument-2 in one intrinsic function.
If a duration is added to the date, and the result is invalid, the date is adjusted. For example, if duration of 1 month is added to the date March 31, 1997, the result would be the invalid date April 31, 1997. This date would be adjusted to the valid date of April 30, 1997.
Example:
Adding months to a specific Date. MOVE FUNCTION ADD-DURATION (date-3 MONTHS 1)TO date-2. MOVE FUNCTION ADD-DURATION (date-3 MONTHS int-1 * 2) TO date-1. Adding years, months, and days to a specific Date MOVE FUNCTION ADD-DURATION (date-1 YEARS 1 MONTHS 5 DAYS 23) TO date-2.
SUBTRACT DURATION – COBOL Function
- The SUBTRACT-DURATION function subtracts a duration from a date, time, or timestamp item, and returns the modified item.
- The function type is date-time.
- The length of the return value depends on the length of the date, time, or timestamp item specified in argument-1. The returned value will be truncated to the length of argument-1.
If a duration is subtracted from a date item, the date returned must fall within a certain range:
- For 4-digit dates, the range must be 0001/01/01 through 9999/12/31
- For 2-digit dates, the range must be 0001/01/01 through 9999/12/31, but the year is truncated to 2 digits
- For a 3-digit year (a 1-digit century and a 2-digit year), the range must be 1900/01/01 through 2899/12/31 (the default). This range can be changed by specifying the DATTIM PROCESS statement option.
If a duration is subtracted from a 2-digit date item, the range is the same as for a 4-digit year, but the year in the value returned is truncated to 2 digits.
SUBTRACT DURATION Format
FUNCTION SUBTRACT-DURATION (argument-1 argument-2 argument-3)
argument-1
- Must be a date, time, or timestamp item.
- Argument-1 is the value from which duration is subtracted. The duration is specified in argument-2 and argument-3.
argument-2
Argument-2 is a keyword that represents a duration. The valid durations are:
- YEARS
- MONTHS
- DAYS
- HOURS
- MINUTES
- SECONDS
- MICROSECONDS
- PICOSECONDS
The duration keyword or conversion specifier used must be consistent with argument-1. For example, the duration keywords must obey the following rules:
- YEARS, MONTHS, and DAYS can only be subtracted from a date or timestamp item.
- HOURS, MINUTES, SECONDS, and MICROSECONDS can only be subtracted from a time or timestamp item.
- PICOSECONDS can only be subtracted from a timestamp item.
argument-3
- Must be an integer arithmetic expression. Argument-3 is the number of units of the duration, as specified in argument-2, that is to be subtracted from argument-1.
- Argument-2 and argument-3 can be repeated. There should be no duplicate argument-2 in one intrinsic function.
- Argument-3 can be a negative integer, but the function only takes its absolute value. If argument-3 is longer than 9 digits, it is truncated.
If a duration is subtracted from a date, and the result is invalid, the date is adjusted. For example, if the duration of 1 month is subtracted from the date May 31, 1997, the result would be the invalid date April 31, 1997. This date would be adjusted to the valid date of April 30, 1997.
Example:
Adding months to a specific Date. MOVE FUNCTION SUBTRACT-DURATION (date-1 MONTHS 1) TO date-2. MOVE FUNCTION SUBTRACT-DURATION (date-2 MONTHS 1 + 2 * 3) TO date-1. Adding years, months, and days to a specific Date MOVE FUNCTION SUBTRACT-DURATION (date-3 MONTHS 5 DAYS 1000) TO date-1.
MOD – COBOL Function
The MOD function returns an integer value that is argument-1 modulo argument-2.
The function type is an integer.
The function result is an integer with as many digits as the shorter of argument-1 and argument-2.
MOD Format
FUNCTION MOD (argument-1 argument-2)
argument-1
Must be an integer.
argument-2
- Must be an integer. Must not be zero.
- The returned value is argument-1 modulo argument-2. The returned value is defined as:
- argument-1 – (argument-2 * FUNCTION INTEGER (argument-1 / argument-2))
The following illustrates the expected results for some values of argument-1 and argument-2.
Example
Argument-1 | Argument-2 | Return |
11 | 5 | 1 |
-11 | 5 | 4 |
11 | -5 | -4 |
-11 | -5 | -1 |
Example: Subtract the specified number of months from an input date.
01 MONTH-END-DD PIC X(24) VALUE '312831303130313130313031'. 01 TBL-MONTH-END REDEFINES MONTH-END-DD. Â Â 03 TBL-MONTH-END-DAY OCCURS 12 TIMES. Â Â Â 05 TBL-MONTH-END-DD PIC 9(02). 01 WS-DATE. Â Â 02 YYYY PIC 9(4). Â Â 02 MM PIC 99. Â Â 02 DD PIC 99. MOVE INPUT-DATE TO WS-DATE DIVIDE WS-NO-OF-MONTHS BY 12 GIVING WS-NO-OF-YEARS Â Â Â Â REMAINDER WS-REMAINING-MONTHS SUBTRACT WS-NO-OF-YEARS FROM YYYY IF MM > WS-REMAINING-MONTHS Â Â COMPUTE MM = MM - WS-REMAINING-MONTHS ELSE Â Â SUBTRACT 1 FROM YYYY Â Â COMPUTE MM = 12 - WS-REMAINING-MONTHS END-IF IF ( FUNCTION MOD (YYYY, 400) = 0) OR Â Â ( FUNCTION MOD (YYYY, 4) = 0 AND Â Â Â FUNCTION MOD (YYYY, 100) NOT = 0 ) Â Â Â MOVE '29' TO TBL-MONTH-END-DD (3:2) ELSE Â Â Â MOVE '28' TO TBL-MONTH-END-DD (3:2) END-IF IF DD > TBL-MONTH-END-DAY (MM) Â Â MOVE TBL-MONTH-END-DAY (MM) TO DD END-IF
Conclusion
Mastering duration calculations in COBOL is crucial for handling date and time operations efficiently, especially in applications that deal with financial transactions and mainframe systems. By understanding how to find, add, subtract, and perform modular arithmetic on durations, COBOL developers can enhance their ability to work with temporal data effectively.