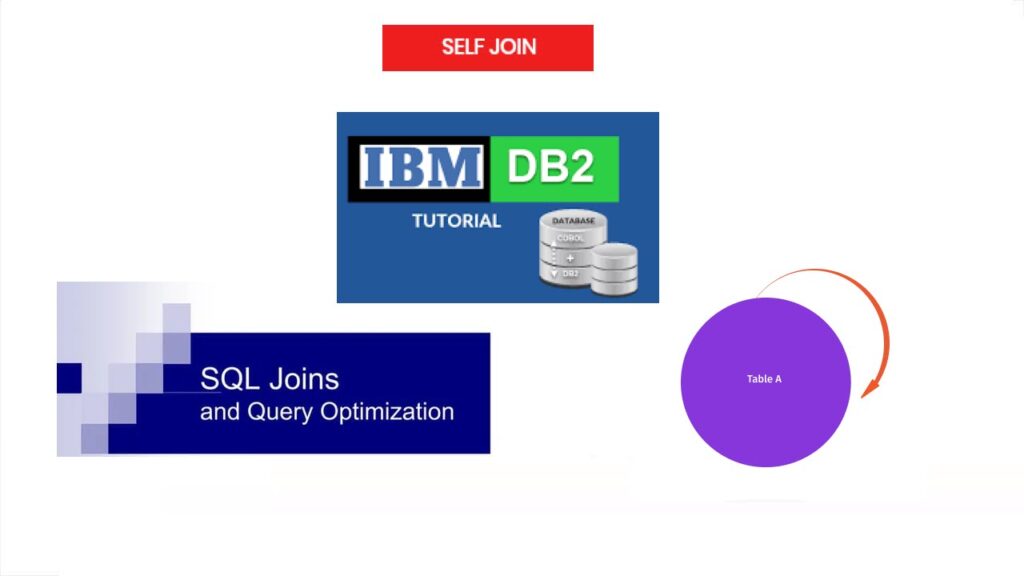
Self-Join in DB2 is a process of joining a table to itself. This is done by giving the table two different aliases and then joining these aliases on a specified column. Self-joins are helpful when you want to compare a row with other rows in the same table.
For example, let’s say you have an employees table with columns: “id”, “name”, and “manager_id”. A self-join on this table using the “id” and “manager_id” columns can be used to create a hierarchy of employees and their managers.
Here’s a simple example of a self-joins in DB2:
SELECT e1.name as employee, e2.name as manager FROM employees e1 JOIN employees e2 ON e1.manager_id = e2.id
This query will return a result set that shows the relationship between employees and their managers.
It’s important to note that self-joins can have performance implications, particularly on larger tables. To avoid slow performance, use appropriate indexes and limit the result set to only the necessary columns.
Advantages and Disadvantages of Self-Join
Advantages of Self-Join:
- It allows for complex relationships to be defined within a single table, making managing and maintaining data easier.
- It can be used to find hierarchical relationships within data, such as employee-manager relationships.
- It can also be used to identify duplicate data or find patterns in data.
Disadvantages of Self-Join:
- Performance issues can arise with large data sets, making the query slow to execute.
- The self-joins can become complex and difficult to understand, especially for complex relationships.
- There is an increased risk of data duplication and incorrect relationships if the data is not properly managed.
Performance improvement for Self-Join
Here are a few ways to improve the performance of a self-joins:
- Use appropriate indexes: Make sure that the columns used in the self-join have an index. This will help the database quickly find the matching rows.
- Limit the result set: Only select the necessary columns in the result set. Avoid using “SELECT *”.
- Use proper join conditions: Ensure that the join condition is as specific as possible to minimize the number of rows that need to be processed.
- Use subqueries: If possible, use subqueries to reduce the number of rows processed by the self-join.
- Use optimized database systems: Consider using an optimized database system that is designed to handle complex queries.
- Consider alternative solutions: In some cases, it may be possible to refactor the data or use alternative solutions to achieve the desired results without using a self-join.
Example of Self-Join
Consider a sample data table called “employees” with columns “id”, “name”, and “manager_id”. The table contains the following data:
-------------------------------- id | name | manager_id -------------------------------- 1 | John Doe | 3 2 | Jane Doe | 3 3 | Bob Smith | 4 4 | Alice Smith| null
Here’s an example of a self-join on the “employees” table to find the manager for each employee:
SELECT e1.name as employee, e2.name as manager FROM employees e1 JOIN employees e2 ON e1.manager_id = e2.id
The result of the self-join query would be:
--------------------- employee | manager --------------------- John Doe | Bob Smith Jane Doe | Bob Smith Bob Smith | Alice Smith
In this example, the self-join allows us to find the manager for each employee by joining the “employees” table to itself using the “manager_id” column. The first alias “e1” represents the employee and the second alias “e2” represents the manager.
Advanced Example of Self-Join
Here’s an advanced example of a self-join with sample data. Consider a sample data table called “employees” with columns “id”, “name”, “salary”, and “manager_id”. The table contains the following data:
----------------------------------------- id | name | salary | manager_id ----------------------------------------- 1 | John Doe | 50000 | 3 2 | Jane Doe | 55000 | 3 3 | Bob Smith | 60000 | 4 4 | Alice Smith| 65000 | null 5 | Tom Johnson| 45000 | 1 6 | Bob Johnson| 40000 | 2
Here’s an example of a self-join to find the average salary for employees and their direct subordinates:
SELECT e1.name as employee, AVG(e2.salary) as avg_subordinate_salary FROM employees e1 JOIN employees e2 ON e1.id = e2.manager_id GROUP BY e1.name
The result of this self-join query would be:
----------------------------------------- employee | avg_subordinate_salary ----------------------------------------- Bob Smith | 47500 John Doe | 52500 Jane Doe | 45000
In this example, the self-join is used to find the average salary for employees and their direct subordinates. The query joins the “employees” table to itself using the “manager_id” column, which represents the relationship between the employee and their direct subordinate. The result set shows the average salary for each employee and their subordinates, grouped by the employee’s name.
Conclusion
A self-join is a regular join, but the table is joined with itself. It allows you to compare rows within the same table by combining records with matching values in a new result set. This can be useful for data analysis and manipulation tasks, such as finding pairs of rows with similar values or generating hierarchical data structures.