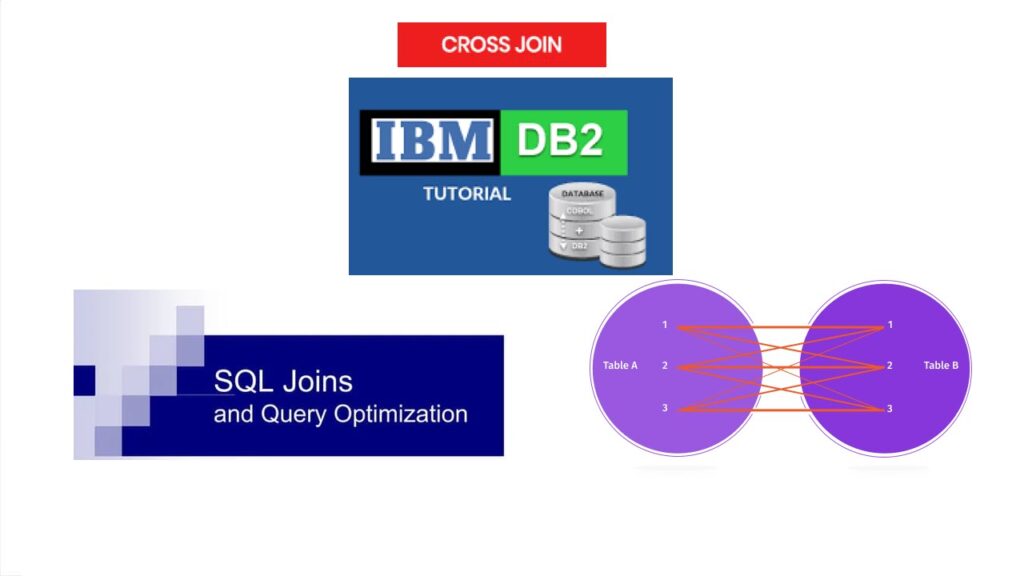
A Cross-Join, also known as a cartesian product, is a type of join operation that returns the combination of every row from one table with every row from another table. It generates all possible combinations of rows between two tables, resulting in a large number of rows in the result set.
In DB2, a Cross-Join is performed using the CROSS JOIN clause in a SELECT statement. The syntax for a cross-join in DB2 is:
SELECT [columns] FROM table1 CROSS JOIN table2; SELECT products.product_id, products.product_name, regions.region_id, regions.region_name FROM products CROSS JOIN regions;
Explanation:
- Selection of Columns: In the
SELECT
clause, we choose specific columns from both tables that we want to include in the result set. - Table Specification: We specify the tables (
products
andregions
) involved in the cross-join operation in theFROM
clause. - CROSS JOIN Operation: The
CROSS JOIN
keyword is used to generate the Cartesian product of the two tables, creating all possible combinations of products and regions. - Result Set: The result set includes columns from both tables, showcasing each product’s interaction with every region.
The CROSS JOIN clause must be used between the two tables that are being joined, and the columns from each table that should be included in the result set can be specified in the SELECT clause.
It is important to note that a cross-join can result in a very large number of rows, so it should be used with caution, especially when joining large tables. To reduce the size of the result set, it is often necessary to include a WHERE clause to filter the rows based on specific conditions.
In summary, a cross-join in DB2 is a type of join operation that returns the combination of every row from one table with every row from another table. It is performed using the CROSS JOIN clause and can result in a large number of rows in the result set.
Advantages of Cross-Join in DB2
- Simplicity: A cross-join is a simple way to combine all rows from two or more tables into a single result set without any specific condition or criteria.
- Complete Data: Cross-join provides complete data by combining all the data from each table, resulting in a comprehensive data set.
- Easy to understand: Cross-join is easy to understand and does not require a complex understanding of join conditions or criteria.
Disadvantages of Cross-Join in DB2
- Performance: Cross-join can be very resource-intensive and slow, especially when joining large tables, resulting in a significant reduction in performance.
- Data Overload: Cross-join generates all possible combinations of rows between two tables, resulting in a large number of rows in the result set, which can cause data overload and lead to performance issues.
- Unwanted Data: Cross-join may result in unwanted data as it combines all the data from each table, including redundant or irrelevant data.
In conclusion, cross-join has both advantages and disadvantages. It can provide complete data and is easy to understand, but can be resource-intensive and slow, resulting in data overload and unwanted data. It is essential to use cross-join wisely, considering the size of the tables being joined and the purpose of the result set.
Performance improvement for Cross-Join
Here are some tips to improve the performance of cross-joins in DB2:
- Use Indexes: Creating indexes on columns used in the join condition can improve the performance of cross-joins. This helps the database quickly identify the matching rows between the two tables.
- Limit the Result Set: Using the LIMIT or FETCH FIRST clause to limit the number of rows in the result set can reduce the amount of data processed, improving performance.
- Use Filters: Adding filters to the join condition can reduce the number of rows in the result set, improving performance.
- Reduce the Size of Tables: Reducing the size of the tables being joined can improve performance by reducing the amount of data that needs to be processed. This can be achieved by removing redundant or irrelevant data.
- Use Temporary Tables: Creating temporary tables with the necessary data and using them in the join can improve performance. This reduces the amount of data that needs to be processed, making the join operation more efficient.
- Use Hash Join: A hash join is a type of join operation that uses a hash table to match rows between the two tables. This type of join can be faster than a cross-join for large tables.
In conclusion, to improve the performance of cross-joins in DB2, it is essential to use indexes, limit the result set, add filters, reduce the size of the tables, use temporary tables, and consider using a hash join.
Example of Cross-Join
Here’s an example of a cross-join in DB2: Suppose we have two tables, “customers” and “orders”, as follows:
Customers: +------------+-------+ | CustomerID | Name | +------------+-------+ | 1 | John | | 2 | Jane | | 3 | Sarah | +------------+-------+ Orders: +---------+------------+---------+ | OrderID | CustomerID | Amount | +---------+------------+---------+ | 1001 | 1 | 200.00 | | 1002 | 2 | 150.00 | | 1003 | 1 | 300.00 | +————+------------+---------+
To perform a cross-join between these two tables, we can use the following SQL statement:
SELECT customers.CustomerID, customers.Name, orders.OrderID, orders.Amount FROM customers CROSS JOIN orders;
The result of this cross-join would be:
+------------+-------+---------+---------+ | CustomerID | Name | OrderID | Amount | +------------+-------+---------+---------+ | 1 | John | 1001 | 200.00 | | 1 | John | 1002 | 150.00 | | 1 | John | 1003 | 300.00 | | 2 | Jane | 1001 | 200.00 | | 2 | Jane | 1002 | 150.00 | | 2 | Jane | 1003 | 300.00 | | 3 | Sarah | 1001 | 200.00 | | 3 | Sarah | 1002 | 150.00 | | 3 | Sarah | 1003 | 300.00 | +------------+-------+---------+---------+
In this example, the cross-join combines every row from the “customers” table with every row from the “orders” table, resulting in a large number of rows in the result set.
Here’s an advanced example of a cross-join in DB2: Suppose we have three tables, “products”, “colors”, and “sizes”, as follows:
Products: +----+---------+ | ID | Product | +----+---------+ | 1 | Shoes | | 2 | Shirt | | 3 | Pants | +----+---------+ Colors: +----+-------+ | ID | Color | +----+-------+ | 1 | Red | | 2 | Blue | | 3 | Green | +----+-------+ Sizes: +----+-----+ | ID | Size | +----+-----+ | 1 | S | | 2 | M | | 3 | L | +----+-----+
To perform a cross-join between these three tables, we can use the following SQL statement:
SELECT products.Product, colors.Color, sizes.Size FROM products CROSS JOIN colors CROSS JOIN sizes;
The result of this cross-join would be:
+---------+-------+-----+ | Product | Color | Size | +---------+-------+-----+ | Shoes | Red | S | | Shoes | Red | M | | Shoes | Red | L | | Shoes | Blue | S | | Shoes | Blue | M | | Shoes | Blue | L | | Shoes | Green | S | | Shoes | Green | M | | Shoes | Green | L | | Shirt | Red | S | | Shirt | Red | M | | Shirt | Red | L | | Shirt | Blue | S | | Shirt | Blue | M | | Shirt | Blue | L | | Shirt | Green | S | | Shirt | Green | M | | Shirt | Green | L | | Pants | Red | S | | Pants | Red | M | | Pants | Red | L | | Pants | Blue | S | | Pants | Blue | M | | Pants | Blue | L | | Pants | Green | S | | Pants | Green | M | | Pants | Green | L | +---------+-------+-----+
In this example, the cross-join combines every row from the “products” table with every row from the “colors” table and every row from the “sizes” table, resulting in a large number of rows in the result set. This is useful for generating a list of all possible combinations of products, colors, and sizes.
Conclusion
Cross-Joins in DB2 is a type of join operation that combines every row from one table with every row from another table, resulting in a Cartesian product. They are often used for testing and debugging purposes or for generating a combination of all possible combinations of data. However, they can also be a source of performance issues if used excessively, as they can produce many rows and consume a significant amount of memory. Therefore, it’s important to use cross-joins judiciously and consider alternative join methods when possible.