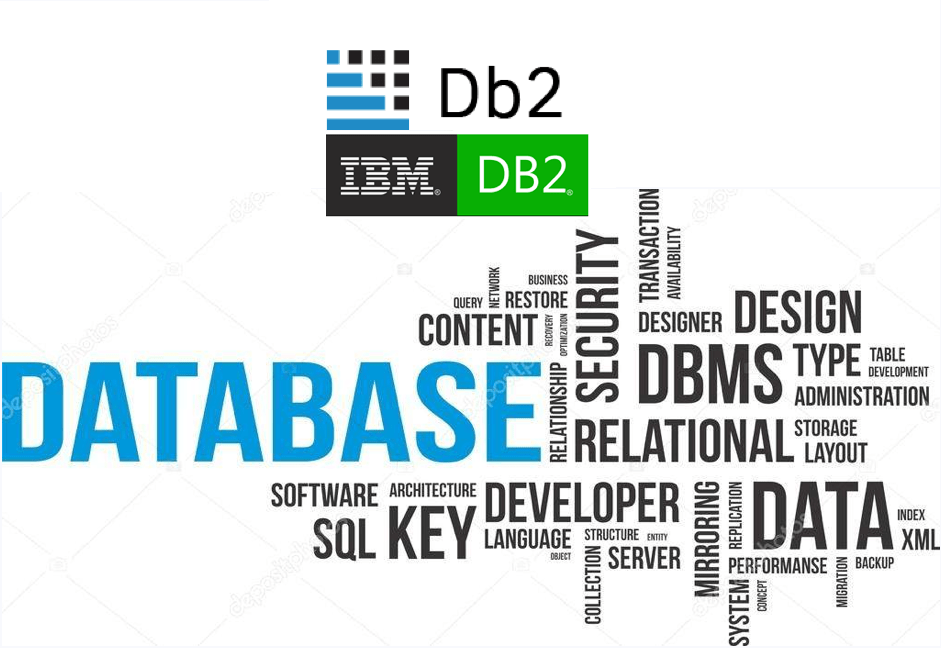
A Subquery Join is a combination of a subquery and a join operation in a SQL query. It allows you to retrieve data from multiple tables in a relational database by combining the results of a subquery with the results of a regular join. The subquery is executed first and its results are used as input to the join operation. This allows you to filter data in one table based on conditions specified in another table. The results of the subquery join are then returned as the final result of the query.
Subquery Joins are useful for retrieving complex information about relationships between tables and can simplify complex queries by breaking them down into smaller, more manageable parts. However, they can also add complexity to a query and slow down performance if not used carefully. A subquery join combines data from two or more tables by using a subquery, which is a SELECT statement nested within another statement.
There are several types of Subquery Joins:
- INNER JOIN: Returns only matching rows from both tables.
- OUTER JOIN: Returns all rows from one table and the matching rows from the other table. There are three types of outer joins: LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN.
- CROSS JOIN: Returns the Cartesian product of the two tables, which is every possible combination of rows from each table.
Advantages of Subquery Join
- Flexibility: Subquery joins allow for complex data relationships to be queried and displayed in a single result set.
- Improved performance: Subqueries can be used to optimize the performance of complex queries by breaking them down into smaller, more manageable components.
- Simplification: Subquery joins can simplify complex queries and make them easier to understand and maintain.
- Reusability: Subqueries can be used as reusable components in multiple queries, reducing the need for duplicated code.
Disadvantages of Subquery Join
- Performance: Subqueries can slow down the performance of a query if not optimized correctly, especially for large data sets.
- Complexity: Subqueries can make a query more complex and difficult to understand, especially for inexperienced users.
- Maintenance: Subqueries can make it more difficult to maintain a query, as changes made to the subquery will affect all queries that use it.
- Limitations: Subqueries have certain limitations in terms of the types of data that can be queried and the ways in which data can be displayed.
Overall, subquery joins offer several advantages and disadvantages, and it is important to carefully consider their use in specific situations to determine if they are the best solution for a given problem.
Performance improvement for Subquery Join
To improve the performance of subquery joins, consider the following tips:
- Indexing: Create indexes on columns used in the join conditions to improve query performance.
- Order of execution: Consider the order of execution of the subquery and main query to ensure that the subquery returns the minimum necessary data.
- Reduce data: Use filtering conditions in the subquery to return only the necessary data, reducing the amount of data that needs to be processed by the main query.
- Temporary tables: Consider using temporary tables to store the results of the subquery, rather than executing the subquery repeatedly in the main query.
- Use appropriate join type: Choose the appropriate type of join (INNER, OUTER, CROSS) based on the requirements of the query.
- Avoid using correlated subqueries: Correlated subqueries can be slow, as they require re-execution for each row processed by the main query. Consider alternative solutions, such as a regular join or an aggregate function.
- Use EXISTS instead of IN: When checking for the existence of related records, use the EXISTS operator instead of the IN operator, as EXISTS is more efficient.
- Avoid using complex expressions: Avoid using complex expressions in the join conditions, as they can slow down query performance.
- Use derived tables: Consider using derived tables (also known as inline views) to store the results of the subquery, making the main query simpler and easier to understand.
- Use hints: If necessary, use hints to control the execution plan of the query and improve performance.
- Parallel execution: Consider using parallel execution to split the processing of a subquery join across multiple cores or processors, improving query performance.
- Avoid subqueries in the SELECT statement: Avoid using subqueries in the SELECT statement, as they can be slow and negatively impact performance.
- Avoid using subqueries in sorting and grouping operations: Avoid using subqueries in sorting and grouping operations, as they can slow down performance.
- Avoid nested subqueries: Avoid using nested subqueries, as they can add significant overhead to a query and slow down performance.
Example of Subquery Join
Suppose we have two tables: Orders and Customers. The Orders table contains information about customer orders, including the order ID, customer ID, and order date. The Customers table contains information about the customers, including the customer ID, name, and address.
We want to retrieve the names of customers who have made orders in the last 30 days. We can use a subquery join to accomplish this as follows:
SELECT c.name FROM Customers c WHERE EXISTS ( SELECT 1 FROM Orders o WHERE o.customer_id = c.customer_id AND o.order_date >= DATEADD(day, -30, GETDATE()) )
The subquery (in the EXISTS clause) retrieves the orders made in the last 30 days, and the main query retrieves the names of customers who made orders in that period. This is an example of a subquery join that uses the EXISTS operator.
Suppose we have two tables: Orders and Order Details. The Orders table contains information about customer orders, including the order ID, customer ID, and order date. The Order Details table contains information about the items in each order, including the order ID, product ID, and quantity.
Orders table: +---------+-----------+------------+ | OrderID | CustomerID| Order_Date | +---------+-----------+------------+ | 1 | 1 | 2022-01-01| | 2 | 2 | 2022-02-01| | 3 | 1 | 2022-03-01| | 4 | 2 | 2022-04-01| +---------+-----------+------------+ OrderDetails table: +---------+----------+----------+ | OrderID | ProductID| Quantity | +---------+----------+----------+ | 1 | 123| 15| | 1 | 124| 5| | 2 | 123| 5| | 3 | 124| 15| | 4 | 123| 20| +---------+----------+----------+ Customers table: +-----------+---------+---------+ | CustomerID| Name | Address | +-----------+---------+---------+ | 1 | John | USA | | 2 | Sarah | UK | +-----------+---------+---------+
We want to retrieve the names of customers who have made orders containing more than 10 units of product 123. We can use a subquery join to accomplish this as follows:
SELECT DISTINCT c.Name FROM Customers c JOIN Orders o ON c.CustomerID = o.CustomerID WHERE EXISTS ( SELECT 1 FROM OrderDetails d WHERE d.OrderID = o.OrderID AND d.ProductID = 123 AND d.Quantity > 10 )
The output will be:
+---------+ | Name | +---------+ | John | | Sarah | +---------+
The subquery (in the EXISTS clause) retrieves orders containing more than 10 units of product 123, and the main query retrieves the names of customers who have made these orders. This is an example of a subquery join that uses the EXISTS operator and combines a subquery with a regular join.
Conclusion
In conclusion, subquery joins are a powerful tool for retrieving data from multiple tables in a relational database. They allow you to combine the results of a subquery with the results of a regular join to retrieve complex information about the relationships between tables. Subquery joins have several advantages, including improved performance, readability, and flexibility. They can be used to simplify complex queries and make them easier to understand. However, they also have some disadvantages, including increased complexity and the possibility of slower performance if not used correctly.
Overall, subquery joins can be a valuable tool in your arsenal when working with relational databases, but it’s important to carefully consider their advantages and disadvantages and choose the right approach for each use case.