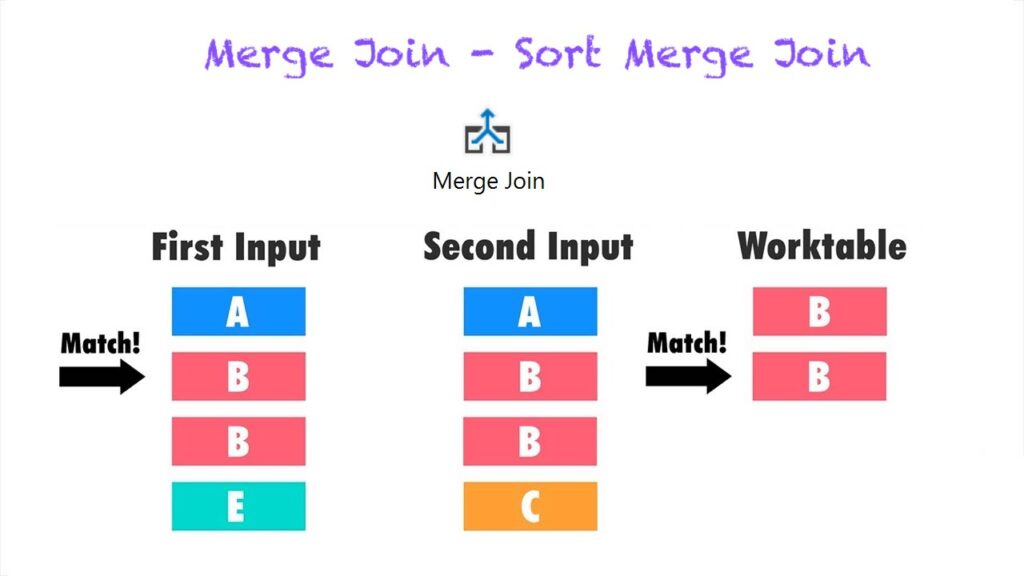
To perform a merge join in DB2, you can use the JOIN keyword in a SELECT statement along with the ON clause to specify the join column. For example, the following query performs a merge join on table1 and table2, using the column as the join column:
SELECT * FROM table1 JOIN table2 ON table1.column = table2.column
It is important to note that the merge join is not the default join method in DB2, it will only work when the tables are already sorted on the join column. If the tables are not sorted, other JOIN methods such as nested loop join or hash join will be used which might not be as efficient.
Performance refinement for MERGE JOIN in DB2
There are several ways to improve the performance of a merge join in DB2:
- Sort the tables: Make sure both tables are already sorted on the join column, or create an index on the join column that can be used to sort the table.
- Use the right data types: Use appropriate data types for the join column to ensure optimal performance.
- Use the right join type: Use the right join type for your queries, such as INNER JOIN or OUTER JOIN.
- Limit the number of columns: Limit the number of columns returned in the query to only the necessary columns.
- Use predicate pushdown: Use predicate pushdown to evaluate the join conditions as early as possible, reducing the amount of data that needs to be processed.
- Use the right join order: Use the right join order, join the table with the smallest number of rows first.
- Use the right buffer pool: Using the right buffer pool for the join tables will help reduce the disk I/O and improve the performance.
- Use parallelism: Using parallelism to split the work across multiple processors will help improve performance, especially when working with large tables.
- Use Explain plan: Use the EXPLAIN PLAN statement to analyze the performance of the query and identify any potential issues.
It’s important to note that these are general recommendations and the performance of the merge join can be affected by many factors such as the size of the tables, the number of rows, the complexity of the query, and the system resources available. It’s always a good idea to test and measure the performance of the query and make adjustments as necessary.
Example of MERGE JOIN
orders
order_id | customer_id | product_id | order_date |
1 | 1 | 101 | 2022-01-01 |
2 | 2 | 102 | 2022-01-02 |
3 | 3 | 103 | 2022-01-03 |
customers
name | name | address |
1 | John Smith | 123 Main St |
2 | Jane Doe | 456 Park Ave |
3 | Bob Johnson | 789 Elm St |
products
product_id | product_name | price |
101 | Computer | 999.99 |
102 | Tablet | 399.99 |
103 | Smartphone | 799.99 |
promotions
promotion_id | product_id | start_date | end_date | discount |
1 | 101 | 2022-01-01 | 2022-01-31 | 0.1 |
2 | 102 | 2022-02-01 | 2022-02-28 | 0.2 |
SELECT orders.order_id, customers.name, products.product_name, products.price, promotions.discount FROM orders JOIN customers ON orders.customer_id = customers.customer_id JOIN products ON orders.product_id = products.product_id LEFT JOIN promotions ON products.product_id = promotions.product_id AND orders.order_date BETWEEN promotions.start_date AND promotions.end_date ORDER BY orders.order_id;
The above query merges data from the “orders”, “customers”, “products” and “promotions” tables and retrieves the order details with the customer name, product name, price, and discount (if any) in sorted order by order_id.
The result would be:
ORDER_ID NAME PRODUCT_NAME PRICE DISCOUNT 1 John Smith Computer 999.99 0.1 2 Jane Doe Tablet 399.99 0.2 3 Bob Johnson Smartphone 799.99 (null)
The above result is sorted by order_id, and for the first two orders, there is a promotion that is valid for the order date, so the discount is applied to the result. However, for the third order, there is no promotion, so the discount column is null.
SORT-MERGE JOIN
A sort-merge join is a type of join in DB2 that combines the features of both a sort and a merge join. It is used when the data to be joined is not already sorted and cannot be accessed through an index. The basic idea behind a sort-merge join is to first sort the data in both tables on the join column, and then merge the sorted data by comparing the values in the join column for each row. The rows with matching values are returned as the result of the join.
One of the advantages of a sort-merge join is that it can handle large amounts of data and can also be used for joins on non-indexed columns. It is also able to handle situations where the join column has duplicate values.
The main disadvantage of a sort-merge join is that it requires a large amount of disk space to sort the data, and can also be slow for small amounts of data. It also requires additional CPU resources to sort the data before joining.
In DB2, the optimizer automatically determines whether a sort-merge join is the best choice for a query, based on the data distribution and other factors.
Overall, sort-merge join is a useful option to join data when you don’t have indexes on join columns or when the data is not already sorted.
Example of SORT MERGE JOIN
orders
order_id | customer_id | product_id | order_date |
1 | 1 | 101 | 2022-01-01 |
2 | 2 | 102 | 2022-01-02 |
3 | 3 | 103 | 2022-01-03 |
customers
name | name | address |
1 | John Smith | 123 Main St |
2 | Jane Doe | 456 Park Ave |
3 | Bob Johnson | 789 Elm St |
products
product_id | product_name | price |
101 | Computer | 999.99 |
102 | Tablet | 399.99 |
103 | Smartphone | 799.99 |
promotions
promotion_id | product_id | start_date | end_date | discount |
1 | 101 | 2022-01-01 | 2022-01-31 | 0.1 |
2 | 102 | 2022-02-01 | 2022-02-28 | 0.2 |
SELECT orders.order_id, customers.name, products.product_name FROM orders, customers, products WHERE orders.customer_id = customers.customer_id AND orders.product_id = products.product_id ORDER BY orders.customer_id
The above query performs a sort-merge join on three tables: orders, customers, and products. It selects the order id, customer name, and product name from the joined tables, and filters the results by matching the customer_id and product_id between the orders and customers tables, and the orders and products tables, respectively. The query also sorts the results by the customer_id.
The result of the above query would be a table with the following columns: order_id, name, product_name. The rows of the table would contain the details of the orders, along with the corresponding customer name, and product name that matches the conditions specified in the query.
For the sample data given in the example query, the result would be:
ORDER_ID NAME PRODUCT_NAME 1 John Smith Computer 2 Jane Doe Tablet 3 Bob Johnson Smartphone
The query starts by selecting all the rows from the three tables, orders, customers, and products, then it filters the results by matching the customer_id and product_id between the orders and customers tables, and the orders and products tables, respectively. Finally, it sorts the results by the customer_id.
Difference between MERGE JOIN and SORT-MERGE JOIN
Feature | Merge Join | Sort-Merge Join |
Data Pre-requisite | Both tables must be sorted on the join column | Tables do not need to be sorted on the join column |
Disk Space | Less disk space is required | More disk space is required to sort the data |
Speed | Faster for large amounts of data | Slower for small amounts of data |
CPU Resources | Fewer CPU resources are required | More CPU resources are required to sort the data |
Indexes | Can use indexes to access the data | Can be used on non-indexed columns |
Duplicate values | Can handle duplicate values in the join column | Can handle duplicate values in the join column |
Conclusion
In conclusion, the merge join is an efficient and powerful way to combine data from two or more tables in DB2, provided that the tables are already sorted on the join column or there is an index on the join column that can be used to sort the table. It can be a great solution for large tables and can help to improve query performance, reducing the time and resources required to return results.
Sort-merge join is a type of join that first sorts the data on the join column and then merges the sorted data by comparing the values in the join column for each row. It is useful when the data is not already sorted and can’t be accessed through an index.